mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
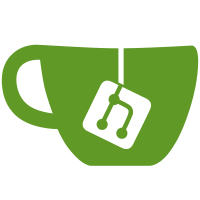
* Fixed duplication kve bug * Autocomplete sort of works now * Even better * Styled autocomplete dropdown * Minor tweaks * Autocomplete looking pretty spiffy * Bug fixes * Apply dropdown to all editors * Fixed key propagation when autocomplete open * Fixed some modals * Split up editor less * Some css improvements * Move filter help out of editor * Fixed drag-n-drop * Perfected autocomplete theme * "fixed" one-line-editor hint click bug * Better autocomplete and switch single line input on drag enter * Don't autocomplete on Tab * Better tag dnd * Add constants completion API * Autocomplete headers * Fixed tests
74 lines
1.9 KiB
JavaScript
74 lines
1.9 KiB
JavaScript
import React, {PropTypes, PureComponent} from 'react';
|
|
import autobind from 'autobind-decorator';
|
|
import KeyValueEditor from '../key-value-editor/editor';
|
|
import {trackEvent} from '../../../analytics/index';
|
|
|
|
@autobind
|
|
class AuthEditor extends PureComponent {
|
|
_handleOnCreate () {
|
|
trackEvent('Auth Editor', 'Create');
|
|
}
|
|
|
|
_handleOnDelete () {
|
|
trackEvent('Auth Editor', 'Delete');
|
|
}
|
|
|
|
_handleToggleDisable (pair) {
|
|
const label = pair.disabled ? 'Disable' : 'Enable';
|
|
trackEvent('Auth Editor', 'Toggle', label);
|
|
}
|
|
|
|
_handleChange (pairs) {
|
|
const pair = {
|
|
username: pairs.length ? pairs[0].name : '',
|
|
password: pairs.length ? pairs[0].value : '',
|
|
disabled: pairs.length ? pairs[0].disabled : false
|
|
};
|
|
|
|
this.props.onChange(pair);
|
|
}
|
|
|
|
render () {
|
|
const {
|
|
authentication,
|
|
showPasswords,
|
|
handleRender,
|
|
handleGetRenderContext
|
|
} = this.props;
|
|
|
|
const pairs = [{
|
|
name: authentication.username || '',
|
|
value: authentication.password || '',
|
|
disabled: authentication.disabled || false
|
|
}];
|
|
|
|
return (
|
|
<KeyValueEditor
|
|
pairs={pairs}
|
|
maxPairs={1}
|
|
disableDelete
|
|
handleRender={handleRender}
|
|
handleGetRenderContext={handleGetRenderContext}
|
|
namePlaceholder="Username"
|
|
valuePlaceholder="•••••••••••"
|
|
valueInputType={showPasswords ? 'text' : 'password'}
|
|
onToggleDisable={this._handleToggleDisable}
|
|
onCreate={this._handleOnCreate}
|
|
onDelete={this._handleOnDelete}
|
|
onChange={this._handleChange}
|
|
/>
|
|
);
|
|
}
|
|
}
|
|
|
|
AuthEditor.propTypes = {
|
|
handleRender: PropTypes.func.isRequired,
|
|
handleGetRenderContext: PropTypes.func.isRequired,
|
|
handleUpdateSettingsShowPasswords: PropTypes.func.isRequired,
|
|
onChange: PropTypes.func.isRequired,
|
|
authentication: PropTypes.object.isRequired,
|
|
showPasswords: PropTypes.bool.isRequired
|
|
};
|
|
|
|
export default AuthEditor;
|