mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
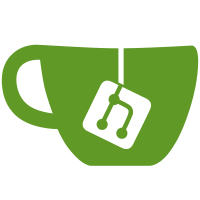
* Fixed duplication kve bug * Autocomplete sort of works now * Even better * Styled autocomplete dropdown * Minor tweaks * Autocomplete looking pretty spiffy * Bug fixes * Apply dropdown to all editors * Fixed key propagation when autocomplete open * Fixed some modals * Split up editor less * Some css improvements * Move filter help out of editor * Fixed drag-n-drop * Perfected autocomplete theme * "fixed" one-line-editor hint click bug * Better autocomplete and switch single line input on drag enter * Don't autocomplete on Tab * Better tag dnd * Add constants completion API * Autocomplete headers * Fixed tests
70 lines
1.6 KiB
JavaScript
70 lines
1.6 KiB
JavaScript
import React, {PropTypes, PureComponent} from 'react';
|
|
import autobind from 'autobind-decorator';
|
|
import Editor from '../codemirror/code-editor';
|
|
import {DEBOUNCE_MILLIS} from '../../../common/constants';
|
|
|
|
@autobind
|
|
class EnvironmentEditor extends PureComponent {
|
|
_handleChange () {
|
|
this.props.didChange();
|
|
}
|
|
|
|
_setEditorRef (n) {
|
|
this._editor = n;
|
|
}
|
|
|
|
getValue () {
|
|
return JSON.parse(this._editor.getValue());
|
|
}
|
|
|
|
isValid () {
|
|
try {
|
|
return this.getValue() !== undefined;
|
|
} catch (e) {
|
|
// Failed to parse JSON
|
|
return false;
|
|
}
|
|
}
|
|
|
|
render () {
|
|
const {
|
|
environment,
|
|
editorFontSize,
|
|
editorKeyMap,
|
|
render,
|
|
getRenderContext,
|
|
lineWrapping,
|
|
...props
|
|
} = this.props;
|
|
|
|
return (
|
|
<Editor
|
|
ref={this._setEditorRef}
|
|
autoPrettify
|
|
fontSize={editorFontSize}
|
|
lineWrapping={lineWrapping}
|
|
keyMap={editorKeyMap}
|
|
onChange={this._handleChange}
|
|
debounceMillis={DEBOUNCE_MILLIS * 6}
|
|
defaultValue={JSON.stringify(environment)}
|
|
render={render}
|
|
getRenderContext={getRenderContext}
|
|
mode="application/json"
|
|
{...props}
|
|
/>
|
|
);
|
|
}
|
|
}
|
|
|
|
EnvironmentEditor.propTypes = {
|
|
environment: PropTypes.object.isRequired,
|
|
didChange: PropTypes.func.isRequired,
|
|
editorFontSize: PropTypes.number.isRequired,
|
|
editorKeyMap: PropTypes.string.isRequired,
|
|
render: PropTypes.func.isRequired,
|
|
getRenderContext: PropTypes.func.isRequired,
|
|
lineWrapping: PropTypes.bool.isRequired
|
|
};
|
|
|
|
export default EnvironmentEditor;
|