mirror of
https://github.com/Kong/insomnia
synced 2024-11-08 14:49:53 +00:00
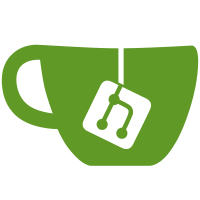
* Update version of react-aria package * Create dropdown hint component * Create dropdown button component * Create the popover component * Create the menu item component * Create the menu section component * Create the menu component * Create the dropdown related components * Change the hotkey html tag * Added full width option for prompt-button * Replace the dropdown with the new * Add some default props for dropdown * Used themed button into dropdown button * Added style prop for dropdown * Remove checkmark from menu item * Added styled props to popover * Remove inner button from account toolbar * Added new dropdown into cookie-list * Revert package update * WIP * Delete button component and replace it's usage with dropdown button * Fix spacing * Added className for code-editor dropdown * Remove the old dropdown * Change import * Revert some ref changes * Added ref to dropdown * Fixed some styles * Fix issues like autosave and added isDisable prop * Add title * Remove insomnia-common imports * Remove insomnia-common imports * Fix code format * Change style and fix code format * Fix styles * Replace dropdown import * Fix divider style * Fix last icon in sidebar create dropdown * Fix issue with dropdown and the resizing screen * Fix linter issues * Moved the prompt button into itemContent component * Change styled component * Fix issue with sidebar-request-row to display the dropdown on hover * Remove the item content in a separate component * Remove unused css * Destructure children props * Fix trigger prop for grpc-method-dropdown * Added prompt button when user delete test suite * Hide empty dropdown items from section * Fix linter issues * Added Checkmark into item content * Fix issue with linter and shortcut component * Fixed linter issues * Revert fragments * Fix linter issues * Added props for multiple select and disable * Fix close issue with sidebar dropdown * Update disable and stay open prop for all dropdowns * Added aria-label for dropdown, section, items * Added selected style for item * Added logic for disabled items * Fixed issue with selected items * Fix issue with selection of grpc method dropdown * Added aria-label to code-editor * Fix issue with onClick and prompt * Hide sections if it's empty * Remove on select from item content * Pass closeOnSelect props in the menu * Removed dataTestId from dropdown items in request-actions * Fix debug sidebar dropdown tests * Change the cookie editor interactions tests * Fix dashboard interactions tests * Fix design-interactions tests * Fixed grpc-interactions tests * Fix plugins interactions tests * Fix preferences interactions tests * Fixed request-pane-tab tests * Fixed app smoke tests * Update git-sync smoke tests * Fix space issue * Fixed graphql smoke test * Fixed oauth smoke test * Fixed oauth smoke test * Fixed websocket smoke test * Fixed cookie-editor-interactions tests * Remove the dropdown unit test * Made small refactoring and remove unnecessary props * Fix issue with grpc-method-dropdown * Change the grpc smoke test * Added default role props * Update all tests that use project role * Remove console.log * Fix issue with themes * REmove dropdown import from index.less file * Remove handleClick and add some comments * Use getItemCount from react-stately * Remove method-dropdown css * Fix shortcut button style * remove extra space on sync-dropdown * fix lint * Remove unused prop * Fix issue with remote workspace dropdown * remove divider on first section if empty * remove unused showGrpc option in method dropdown * Fix issue with workspace-dropdown * Fix git-sync-dropdown issues * Fix issue with remove workspaces dropdown * Fix sync-dropdown component * Added disable style * Moved server reflection and proto file outside of the dropdown * Fix style when item is selected * Fix issue with grpc smoke test * Fix issue with menu props * Disable server reflection button if url is empty Co-authored-by: Filipe Freire <livrofubia@gmail.com> Co-authored-by: gatzjames <jamesgatzos@gmail.com>
75 lines
3.5 KiB
TypeScript
75 lines
3.5 KiB
TypeScript
import { test } from '../../playwright/test';
|
|
|
|
test('Select body dropdown', async ({ page }) => {
|
|
await page.getByRole('button', { name: 'Body' }).click();
|
|
await page.getByRole('menuitem', { name: 'JSON' }).click();
|
|
});
|
|
|
|
test('Select auth dropdown', async ({ page }) => {
|
|
await page.getByRole('tab', { name: 'Auth' }).click();
|
|
await page.getByRole('button', { name: 'Auth' }).click();
|
|
await page.getByRole('menuitem', { name: 'OAuth 1.0' }).click();
|
|
});
|
|
test('Open query parameters', async ({ page }) => {
|
|
await page.getByRole('tab', { name: 'Query' }).click();
|
|
await page.getByRole('tab', { name: 'Headers' }).click();
|
|
});
|
|
|
|
test('Open headers', async ({ page }) => {
|
|
await page.getByRole('tab', { name: 'Headers' }).click();
|
|
});
|
|
|
|
test('Open docs', async ({ page }) => {
|
|
await page.getByRole('tab', { name: 'Docs' }).click();
|
|
});
|
|
|
|
test('Add description to docs', async ({ page }) => {
|
|
await page.getByRole('tab', { name: 'Docs' }).click();
|
|
await page.locator('text=Add Description').click();
|
|
await page.locator('[data-testid="CodeEditor"] pre[role="presentation"]:has-text("")').click();
|
|
await page.locator('textarea').nth(1).fill('new request'); // this works
|
|
// TODO - fix the locator so we don't rely on `.nth(1)` https://linear.app/insomnia/issue/INS-2255/revisit-codemirror-playwright-selectorfill
|
|
});
|
|
|
|
test('WS select body type dropdown', async ({ page }) => {
|
|
await page.locator('[data-testid="SidebarFilter"] [data-testid="SidebarCreateDropdown"] button').click();
|
|
await page.getByRole('menuitem', { name: 'WebSocket Request' }).click();
|
|
await page.getByRole('tab', { name: 'JSON' }).click();
|
|
await page.getByRole('menuitem', { name: 'JSON' }).click();
|
|
});
|
|
|
|
test('WS select auth type dropdown', async ({ page }) => {
|
|
await page.locator('[data-testid="SidebarFilter"] [data-testid="SidebarCreateDropdown"] button').click();
|
|
await page.getByRole('menuitem', { name: 'WebSocket Request' }).click();
|
|
await page.getByRole('tab', { name: 'Auth' }).click();
|
|
});
|
|
|
|
test('WS open query parameters', async ({ page }) => {
|
|
await page.locator('[data-testid="SidebarFilter"] [data-testid="SidebarCreateDropdown"] button').click();
|
|
await page.getByRole('menuitem', { name: 'WebSocket Request' }).click();
|
|
await page.getByRole('tab', { name: 'Query' }).click();
|
|
});
|
|
|
|
test('WS open headers', async ({ page }) => {
|
|
await page.locator('[data-testid="SidebarFilter"] [data-testid="SidebarCreateDropdown"] button').click();
|
|
await page.getByRole('menuitem', { name: 'WebSocket Request' }).click();
|
|
await page.getByRole('tab', { name: 'Headers' }).click();
|
|
});
|
|
|
|
test('WS open docs', async ({ page }) => {
|
|
await page.locator('[data-testid="SidebarFilter"] [data-testid="SidebarCreateDropdown"] button').click();
|
|
await page.getByRole('menuitem', { name: 'WebSocket Request' }).click();
|
|
await page.getByRole('tab', { name: 'Docs' }).click();
|
|
|
|
});
|
|
|
|
test('WS add description', async ({ page }) => {
|
|
await page.locator('[data-testid="SidebarFilter"] [data-testid="SidebarCreateDropdown"] button').click();
|
|
await page.getByRole('menuitem', { name: 'WebSocket Request' }).click();
|
|
await page.getByRole('tab', { name: 'Docs' }).click();
|
|
await page.getByRole('button', { name: 'Add Description' }).click();
|
|
await page.locator('[data-testid="CodeEditor"] pre[role="presentation"]:has-text("")').click();
|
|
await page.locator('textarea').nth(1).fill('new wss');
|
|
// TODO - fix the locator so we don't rely on `.nth(1)` https://linear.app/insomnia/issue/INS-2255/revisit-codemirror-playwright-selectorfill
|
|
});
|