mirror of
https://github.com/Kong/insomnia
synced 2024-11-12 17:26:32 +00:00
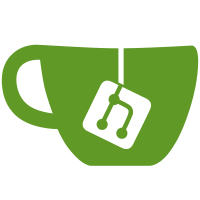
* Fix OAuth token, new request content-type, and more * better error messaging around invalid Curl opts * Make XFERINFOFUNCTION an optional option * Fix content-type change prompt and double quotes in prettify * Don't send Expect header on file upload * Remove dead code * Base64 tag * Fix HTML entities in URL clicks * Fix curl paste
31 lines
983 B
JavaScript
31 lines
983 B
JavaScript
import * as templating from '../../index';
|
|
|
|
function assertTemplate (txt, expected) {
|
|
return async function () {
|
|
const result = await templating.render(txt);
|
|
expect(result).toMatch(expected);
|
|
};
|
|
}
|
|
|
|
function assertTemplateFails (txt, expected) {
|
|
return async function () {
|
|
try {
|
|
await templating.render(txt);
|
|
fail(`Render should have thrown ${expected}`);
|
|
} catch (err) {
|
|
expect(err.message).toBe(expected);
|
|
}
|
|
};
|
|
}
|
|
|
|
describe('Base64EncodeExtension', () => {
|
|
it('encodes nothing', assertTemplate("{% base64 'encode' %}", ''));
|
|
it('encodes something', assertTemplate("{% base64 'encode', 'my string' %}", 'bXkgc3RyaW5n'));
|
|
it('decodes nothing', assertTemplate("{% base64 'decode' %}", ''));
|
|
it('decodes something', assertTemplate("{% base64 'decode', 'bXkgc3RyaW5n' %}", 'my string'));
|
|
it('fails on invalid op', assertTemplateFails(
|
|
"{% base64 'foo' %}",
|
|
'Unsupported operation "foo". Must be encode or decode.'
|
|
));
|
|
});
|