mirror of
https://github.com/nocobase/nocobase
synced 2024-11-16 02:45:14 +00:00
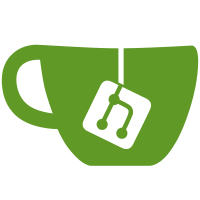
* fix: add field linkage on setting default datetime * fix: fix dateonly timezone problem * fix: improve test * docs(DatePicker): add demos * fix(DatePicker): should return the beginning of a second * feat(DatePicker): support non-UTC * refactor: rename * fix(RangePicker): get correct end date * test(mapDatePicker): add test * test(mapRangePicker): add test * feat(Filter): use non-UTC to filter * feat(FilterBlock): use non-UTC to filter * feat: add '$dateBetween' operator in datetime * feat: use RangePicker on toggled to 'dateBetween' operator * feat: set ranges for RangePicker * feat: backend support to parse 'dateBetween' operator * fix: fix build error * fix: adaptive content width * feat: support to use var on data scope * feat: add parse-variables plugin * feat: support to parse variables * feat: support only to set system variables * test: rename * feat: cover all * fix: fix build error * feat(RangePicker): extend more shortcut keys * feat(parse-variables): support more date var * feat: support user variables * feat: disable unmatched options * fix: use component name to filter option * fix: fix build error * feat: remove some operator of id * chore: remove useless operators * fix: built in plugin * refactor: move to core from plugin * refactor: remove code of plugin * refactor: remove useless code * fix: should after acl * Update server.ts * fix: compatible with old version * feat: test cases * refactor: rename to 'is between' * refactor: parse filter * fix: improve code * feat: test cases * fix: fix error * fix: improve parse date * fix: date variables * fix: day range * fix: test error * fix: typo * fix: test error * feat: $user variable * fix: toDate * fix: fix the value range of shortcuts * feat: add quarter and test * feat: support to use user's association fields to filter * refactor: use maxDepth * refactor: remove useless code * fix: make AssociationSelect.Designer to support variables * fix: getField * fix: parse utc * fix: remove only * fix: filter by ctx.db.getFieldByPath * fix: avoid error * fix: add translation * fix(RangePicker): can be set to empty * feat(utils): add hasEmptyValue * fix: should not save empty * fix: last few days should include today * fix: limit user variable type to display * fix: parse filter error * fix: empty * test: [skip ci] * fix: remove ';' * feat: improve code --------- Co-authored-by: chenos <chenlinxh@gmail.com>
125 lines
3.0 KiB
TypeScript
125 lines
3.0 KiB
TypeScript
import moment from 'moment';
|
|
|
|
export interface Str2momentOptions {
|
|
gmt?: boolean;
|
|
picker?: 'year' | 'month' | 'week' | 'quarter';
|
|
utcOffset?: any;
|
|
utc?: boolean;
|
|
}
|
|
|
|
export const getDefaultFormat = (props: any) => {
|
|
if (props.format) {
|
|
return props.format;
|
|
}
|
|
if (props.dateFormat) {
|
|
if (props['showTime']) {
|
|
return `${props.dateFormat} ${props.timeFormat || 'HH:mm:ss'}`;
|
|
}
|
|
return props.dateFormat;
|
|
}
|
|
if (props['picker'] === 'month') {
|
|
return 'YYYY-MM';
|
|
} else if (props['picker'] === 'quarter') {
|
|
return 'YYYY-\\QQ';
|
|
} else if (props['picker'] === 'year') {
|
|
return 'YYYY';
|
|
} else if (props['picker'] === 'week') {
|
|
return 'YYYY-wo';
|
|
}
|
|
return props['showTime'] ? 'YYYY-MM-DD HH:mm:ss' : 'YYYY-MM-DD';
|
|
};
|
|
|
|
export const toGmt = (value: moment.Moment) => {
|
|
if (!value || !moment.isMoment(value)) {
|
|
return value;
|
|
}
|
|
return `${value.format('YYYY-MM-DD')}T${value.format('HH:mm:ss.SSS')}Z`;
|
|
};
|
|
|
|
export const toLocal = (value: moment.Moment) => {
|
|
if (!value) {
|
|
return value;
|
|
}
|
|
if (Array.isArray(value)) {
|
|
return value.map((val) => val.startOf('second').toISOString());
|
|
}
|
|
if (moment.isMoment(value)) {
|
|
return value.startOf('second').toISOString();
|
|
}
|
|
};
|
|
|
|
const toMoment = (val: any, options?: Str2momentOptions) => {
|
|
if (!val) {
|
|
return;
|
|
}
|
|
const offset = options.utcOffset || -1 * new Date().getTimezoneOffset();
|
|
const { gmt, picker, utc = true } = options;
|
|
|
|
if (!utc) {
|
|
return moment(val);
|
|
}
|
|
|
|
if (moment.isMoment(val)) {
|
|
return val.utcOffset(offset);
|
|
}
|
|
if (gmt || picker) {
|
|
return moment(val).utcOffset(0);
|
|
}
|
|
return moment(val).utcOffset(offset);
|
|
};
|
|
|
|
export const str2moment = (value?: string | string[], options: Str2momentOptions = {}): any => {
|
|
return Array.isArray(value)
|
|
? value.map((val) => {
|
|
return toMoment(val, options);
|
|
})
|
|
: value
|
|
? toMoment(value, options)
|
|
: value;
|
|
};
|
|
|
|
const toStringByPicker = (value, picker) => {
|
|
if (picker === 'year') {
|
|
return value.format('YYYY') + '-01-01T00:00:00.000Z';
|
|
}
|
|
if (picker === 'month') {
|
|
return value.format('YYYY-MM') + '-01T00:00:00.000Z';
|
|
}
|
|
if (picker === 'quarter') {
|
|
return value.format('YYYY-MM') + '-01T00:00:00.000Z';
|
|
}
|
|
if (picker === 'week') {
|
|
return value.format('YYYY-MM-DD') + 'T00:00:00.000Z';
|
|
}
|
|
return value.format('YYYY-MM-DD') + 'T00:00:00.000Z';
|
|
};
|
|
|
|
const toGmtByPicker = (value: moment.Moment | moment.Moment[], picker?: any) => {
|
|
if (!value) {
|
|
return value;
|
|
}
|
|
if (Array.isArray(value)) {
|
|
return value.map((val) => toStringByPicker(val, picker));
|
|
}
|
|
if (moment.isMoment(value)) {
|
|
return toStringByPicker(value, picker);
|
|
}
|
|
};
|
|
|
|
export interface Moment2strOptions {
|
|
showTime?: boolean;
|
|
gmt?: boolean;
|
|
picker?: 'year' | 'month' | 'week' | 'quarter';
|
|
}
|
|
|
|
export const moment2str = (value?: moment.Moment, options: Moment2strOptions = {}) => {
|
|
const { showTime, gmt, picker } = options;
|
|
if (!value) {
|
|
return value;
|
|
}
|
|
if (showTime) {
|
|
return gmt ? toGmt(value) : toLocal(value);
|
|
}
|
|
return toGmtByPicker(value, picker);
|
|
};
|