mirror of
https://github.com/nocobase/nocobase
synced 2024-11-17 01:26:12 +00:00
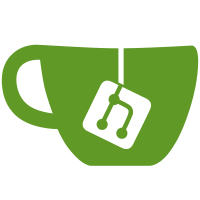
* feat(charts-v2): init * chore(charts-v2): init chart renderer * feat(chart-v2): add chart grid and initializer * feat(chart-v2): improve ui * feat(chart-v2): ui * feat(charts-v2): query sort ui * feat(charts-v2): field select component * feat(charts-v2): improve ui && add query action * feat(charts-v2): imporve ui, work in progress * fix(charts-v2): chart renderer request api twice * feat(charts-v2): add dimension formatter * feat(charts-v2): filter, sort, limit * feat(charts-v2): sql mode ui * feat(charts-v2): support duplicate & sql mode * fix(charts-v2): wrong defaultValue of json config * feat(charts-v2): transformer ui * feat(charts-v2): transformer * chore(charts-v2): rename transfromer to transform * feat(charts-v2): support cache * feat(charts-v2): add acl provider * chore(charts-v2): hide sql mode * refactor(charts-v2): add renderer provider * feat: collection permission check * feat(charts-v2): add antd statistic * test(charts-v2): backend * chore: improve code * test(charts-v2): add test * chore: add Chinese translation * fix(charts-v2): locale switch bug * chore: add dependency * feat(charts-v2): init chart config from query * feat: change layout * test: fix frontend test * feat: improve auto infer * fix: ui issues * chore: translation * fix: sql error * fix: some issues * feat: support table * fix: bug * chore: improve code and fix query * feat: add config reference * chore: add translation * fix: process data due to pg issue * test: fix parseBuilder * chore: upgrade formily to 2.2.25 * fix: some issues and import style * fix: bug when query with sort * feat: parse enum data * fix: yarn.lock * fix: type error * fix: infer bug and frontend test * test: fix frontend * fix: test * feat: improve preview * chore: downgrade formily * feat: support associations, draft, in testing * fix: typo * test: frontend & backend * fix: infer bug * feat: measure selection of statistics * fix: bug of group by alias * fix: some issues * fix: order issues * fix: yarn.lock * chore: fix filter include & 'data-visualization' * style: improve style * docs: add readme * chore: add translation --------- Co-authored-by: chenos <chenlinxh@gmail.com>
105 lines
2.8 KiB
TypeScript
105 lines
2.8 KiB
TypeScript
import { registerValidateRules } from '@formily/core';
|
|
import {
|
|
SchemaComponentOptions,
|
|
SchemaInitializerContext,
|
|
SettingsCenterProvider,
|
|
useAPIClient,
|
|
} from '@nocobase/client';
|
|
import JSON5 from 'json5';
|
|
import React, { useContext } from 'react';
|
|
import { ChartBlockEngine } from './ChartBlockEngine';
|
|
import { ChartBlockInitializer } from './ChartBlockInitializer';
|
|
import { ChartQueryMetadataProvider } from './ChartQueryMetadataProvider';
|
|
import './Icons';
|
|
import { lang } from './locale';
|
|
import { CustomSelect } from './select';
|
|
import { QueriesTable } from './settings/QueriesTable';
|
|
|
|
registerValidateRules({
|
|
json5: (value, rule) => {
|
|
if (!value) {
|
|
return '';
|
|
}
|
|
try {
|
|
const val = JSON5.parse(value);
|
|
if (!isNaN(val)) {
|
|
return {
|
|
type: 'error',
|
|
message: lang('Invalid JSON format'),
|
|
};
|
|
}
|
|
return '';
|
|
} catch (error) {
|
|
console.error(error);
|
|
return {
|
|
type: 'error',
|
|
message: lang('Invalid JSON format'),
|
|
};
|
|
}
|
|
},
|
|
});
|
|
|
|
const Charts = React.memo((props) => {
|
|
const api = useAPIClient();
|
|
const items = useContext<any>(SchemaInitializerContext);
|
|
const children = items.BlockInitializers.items[0].children;
|
|
if (children) {
|
|
const hasChartItem = children.some((child) => child?.component === 'ChartBlockInitializer');
|
|
if (!hasChartItem) {
|
|
children.push({
|
|
key: 'chart',
|
|
type: 'item',
|
|
icon: 'PieChartOutlined',
|
|
title: '{{t("Chart (Old)",{ns:"charts"})}}',
|
|
component: 'ChartBlockInitializer',
|
|
});
|
|
}
|
|
}
|
|
const validateSQL = (sql) => {
|
|
return new Promise((resolve) => {
|
|
api
|
|
.request({
|
|
url: 'chartsQueries:validate',
|
|
method: 'post',
|
|
data: {
|
|
sql,
|
|
},
|
|
})
|
|
.then(({ data }) => {
|
|
resolve(data?.data?.errorMessage);
|
|
})
|
|
.catch(() => {
|
|
resolve('Invalid SQL');
|
|
});
|
|
});
|
|
};
|
|
return (
|
|
<ChartQueryMetadataProvider>
|
|
<SettingsCenterProvider
|
|
settings={{
|
|
charts: {
|
|
title: '{{t("Charts", {ns:"charts"})}}',
|
|
icon: 'PieChartOutlined',
|
|
tabs: {
|
|
queries: {
|
|
title: '{{t("Queries", {ns:"charts"})}}',
|
|
component: QueriesTable,
|
|
},
|
|
},
|
|
},
|
|
}}
|
|
>
|
|
<SchemaComponentOptions
|
|
scope={{ validateSQL }}
|
|
components={{ CustomSelect, ChartBlockInitializer, ChartBlockEngine }}
|
|
>
|
|
<SchemaInitializerContext.Provider value={items}>{props.children}</SchemaInitializerContext.Provider>
|
|
</SchemaComponentOptions>
|
|
</SettingsCenterProvider>
|
|
</ChartQueryMetadataProvider>
|
|
);
|
|
});
|
|
Charts.displayName = 'Charts';
|
|
|
|
export default Charts;
|