mirror of
https://github.com/nocobase/nocobase
synced 2024-11-17 00:50:26 +00:00
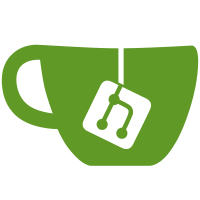
* chore: use vitest to replace jest * chore: support vitest * feat: vitest 1.0 * fix: test * chore: yarn.lock * chore: github actions * fix: test * fix: test * fix: test * fix: test * fix: jest.fn * fix: require * fix: test * fix: build * fix: test * fix: test * fix: test * fix: test * fix: test * fix: test * fix: test * fix: dynamic import * fix: bug * chore: yarn run test command * chore: package.json * chore: package.json * chore: vite 5 * fix: fix variable test * fix: import json * feat: initEnv * fix: env.APP_ENV_PATH * chore: get package json * fix: remove GlobalThmeProvider * chore: update snap * chore: test env * chore: test env * chore: import module * chore: jest * fix: load package json * chore: test * fix: bug * chore: test * chore: test * chore: test * chore: test * chore: test * fix: import file in windows * chore: import module with absolute file path * fix: test error * test: update snapshot * chore: update yarn.lock * fix: front-end tests do not include utils folder * refactor: use vitest-dom * fix: fix build * fix: test error * fix: change to vitest.config.mts * fix: types error * fix: types error * fix: types error * fix: error * fix: test * chore: test * fix: test package * feat: update dependencies * refactor: test * fix: error * fix: error * fix: __dirname is not defined in ES module scope * fix: allow only * fix: error * fix: error * fix: error * fix: create-app * fix: install-deps * feat: update docs --------- Co-authored-by: chenos <chenlinxh@gmail.com> Co-authored-by: dream2023 <1098626505@qq.com> Co-authored-by: Zeke Zhang <958414905@qq.com>
97 lines
2.8 KiB
TypeScript
97 lines
2.8 KiB
TypeScript
import { merge, uid } from '@nocobase/utils';
|
|
import { customAlphabet } from 'nanoid';
|
|
import fetch from 'node-fetch';
|
|
import path, { resolve } from 'path';
|
|
import { Database, IDatabaseOptions } from './database';
|
|
|
|
export class MockDatabase extends Database {
|
|
constructor(options: IDatabaseOptions) {
|
|
super({
|
|
storage: ':memory:',
|
|
dialect: 'sqlite',
|
|
...options,
|
|
});
|
|
}
|
|
}
|
|
|
|
export function getConfigByEnv() {
|
|
const options = {
|
|
username: process.env.DB_USER,
|
|
password: process.env.DB_PASSWORD,
|
|
database: process.env.DB_DATABASE,
|
|
host: process.env.DB_HOST,
|
|
port: process.env.DB_PORT,
|
|
dialect: process.env.DB_DIALECT || 'sqlite',
|
|
logging: process.env.DB_LOGGING === 'on' ? customLogger : false,
|
|
storage: resolve(process.cwd(), `storage/db-test/db-${uid()}.sqlite`),
|
|
define: {
|
|
charset: 'utf8mb4',
|
|
collate: 'utf8mb4_unicode_ci',
|
|
},
|
|
timezone: process.env.DB_TIMEZONE,
|
|
underscored: process.env.DB_UNDERSCORED === 'true',
|
|
schema: process.env.DB_SCHEMA !== 'public' ? process.env.DB_SCHEMA : undefined,
|
|
dialectOptions: {},
|
|
};
|
|
|
|
if (process.env.DB_DIALECT == 'postgres') {
|
|
options.dialectOptions['application_name'] = 'nocobase.main';
|
|
}
|
|
|
|
return options;
|
|
}
|
|
|
|
function customLogger(queryString, queryObject) {
|
|
console.log(queryString); // outputs a string
|
|
if (queryObject.bind) {
|
|
console.log(queryObject.bind); // outputs an array
|
|
}
|
|
}
|
|
|
|
export function mockDatabase(options: IDatabaseOptions = {}): MockDatabase {
|
|
const dbOptions = merge(getConfigByEnv(), options) as any;
|
|
|
|
if (process.env['DB_TEST_PREFIX']) {
|
|
let configKey = 'database';
|
|
if (dbOptions.dialect === 'sqlite') {
|
|
configKey = 'storage';
|
|
} else {
|
|
configKey = 'database';
|
|
}
|
|
|
|
const shouldChange = () => {
|
|
if (dbOptions.dialect === 'sqlite') {
|
|
return !dbOptions[configKey].includes(process.env['DB_TEST_PREFIX']);
|
|
}
|
|
|
|
return !dbOptions[configKey].startsWith(process.env['DB_TEST_PREFIX']);
|
|
};
|
|
|
|
if (dbOptions[configKey] && shouldChange()) {
|
|
const nanoid = customAlphabet('1234567890abcdefghijklmnopqrstuvwxyz', 10);
|
|
|
|
const instanceId = `d_${nanoid()}`;
|
|
const databaseName = `${process.env['DB_TEST_PREFIX']}_${instanceId}`;
|
|
|
|
if (dbOptions.dialect === 'sqlite') {
|
|
dbOptions.storage = path.resolve(path.dirname(dbOptions.storage), databaseName);
|
|
} else {
|
|
dbOptions.database = databaseName;
|
|
}
|
|
}
|
|
|
|
if (process.env['DB_TEST_DISTRIBUTOR_PORT']) {
|
|
dbOptions.hooks = dbOptions.hooks || {};
|
|
|
|
dbOptions.hooks.beforeConnect = async (config) => {
|
|
const url = `http://127.0.0.1:${process.env['DB_TEST_DISTRIBUTOR_PORT']}/acquire?via=${db.instanceId}&name=${config.database}`;
|
|
await fetch(url);
|
|
};
|
|
}
|
|
}
|
|
|
|
const db = new MockDatabase(dbOptions);
|
|
|
|
return db;
|
|
}
|