mirror of
https://github.com/nocobase/nocobase
synced 2024-11-17 18:17:19 +00:00
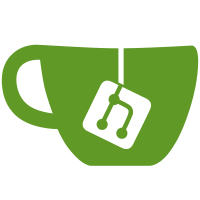
* feat(plugin-formula): add dynamic expression field * feat(plugin-workflow): add dynamic expression for calculation * refactor(client): allow select part of paths in variable component * fix(client): fix types * feat(plugin-formula): add dynamic expression config * feat(plugin-workflow): add dynamic calculation * refactor(plugin-formula): move expression field type to workflow plugin * fix(plugin-workflow): fix types * fix(plugin-workflow): fix register field in client * fix(plugin-workflow): fix expression result value default * fix(plugin-workflow): fix dynamic expression field error when switch collection * fix(plugin-workflow): test component value change * test(plugin-workflow): test component linkages * refactor(plugin-workflow): change to expression collection template * fix(client): fix hooks of Variable.TextArea * fix(client): fix to import evaluators in client * fix(evaluators): move renderReference method to plugin * fix(plugin-workflow): fix missed component * fix(plugin-workflow): fix dynamic expression test case * refactor(client): change popover to double click to choose entire object * refactor(plugin-workflow): make variable options and filter more sensible * fix(plugin-workflow): fix form effect * fix(plugin-workflow): fix variable filtering in collection trigger * fix(plugin-workflow): fix types --------- Co-authored-by: chenos <chenlinxh@gmail.com>
48 lines
1.2 KiB
TypeScript
48 lines
1.2 KiB
TypeScript
import parse from 'json-templates';
|
|
|
|
import evaluators, { Evaluator } from '@nocobase/evaluators';
|
|
|
|
import { Processor } from '..';
|
|
import { JOB_STATUS } from "../constants";
|
|
import FlowNodeModel from "../models/FlowNode";
|
|
import { Instruction } from ".";
|
|
|
|
|
|
|
|
interface CalculationConfig {
|
|
dynamic?: boolean | string;
|
|
engine?: string;
|
|
expression?: string;
|
|
}
|
|
|
|
export default {
|
|
async run(node: FlowNodeModel, prevJob, processor: Processor) {
|
|
const { dynamic = false } = <CalculationConfig>node.config || {};
|
|
let { engine = 'math.js', expression = '' } = node.config;
|
|
let scope = processor.getScope();
|
|
if (dynamic) {
|
|
const parsed = parse(dynamic)(scope) ?? {};
|
|
engine = parsed.engine;
|
|
expression = parsed.expression;
|
|
scope = parse(node.config.scope ?? '')(scope) ?? {};
|
|
}
|
|
|
|
const evaluator = <Evaluator | undefined>evaluators.get(engine);
|
|
|
|
try {
|
|
const result = evaluator && expression
|
|
? evaluator(expression, scope)
|
|
: null;
|
|
return {
|
|
result,
|
|
status: JOB_STATUS.RESOLVED
|
|
};
|
|
} catch (e) {
|
|
return {
|
|
result: e.toString(),
|
|
status: JOB_STATUS.ERROR
|
|
}
|
|
}
|
|
}
|
|
} as Instruction;
|