mirror of
https://github.com/nocobase/nocobase
synced 2024-11-16 01:17:43 +00:00
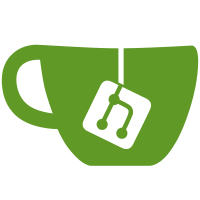
* chore: use vitest to replace jest * chore: support vitest * feat: vitest 1.0 * fix: test * chore: yarn.lock * chore: github actions * fix: test * fix: test * fix: test * fix: test * fix: jest.fn * fix: require * fix: test * fix: build * fix: test * fix: test * fix: test * fix: test * fix: test * fix: test * fix: test * fix: dynamic import * fix: bug * chore: yarn run test command * chore: package.json * chore: package.json * chore: vite 5 * fix: fix variable test * fix: import json * feat: initEnv * fix: env.APP_ENV_PATH * chore: get package json * fix: remove GlobalThmeProvider * chore: update snap * chore: test env * chore: test env * chore: import module * chore: jest * fix: load package json * chore: test * fix: bug * chore: test * chore: test * chore: test * chore: test * chore: test * fix: import file in windows * chore: import module with absolute file path * fix: test error * test: update snapshot * chore: update yarn.lock * fix: front-end tests do not include utils folder * refactor: use vitest-dom * fix: fix build * fix: test error * fix: change to vitest.config.mts * fix: types error * fix: types error * fix: types error * fix: error * fix: test * chore: test * fix: test package * feat: update dependencies * refactor: test * fix: error * fix: error * fix: __dirname is not defined in ES module scope * fix: allow only * fix: error * fix: error * fix: error * fix: create-app * fix: install-deps * feat: update docs --------- Co-authored-by: chenos <chenlinxh@gmail.com> Co-authored-by: dream2023 <1098626505@qq.com> Co-authored-by: Zeke Zhang <958414905@qq.com>
95 lines
1.9 KiB
TypeScript
95 lines
1.9 KiB
TypeScript
import { importModule } from '@nocobase/utils';
|
|
import _ from 'lodash';
|
|
import { QueryInterface, Sequelize } from 'sequelize';
|
|
import Database from './database';
|
|
|
|
export interface MigrationContext {
|
|
db: Database;
|
|
queryInterface: QueryInterface;
|
|
sequelize: Sequelize;
|
|
}
|
|
|
|
export class Migration {
|
|
public name: string;
|
|
|
|
public context: { db: Database; [key: string]: any };
|
|
|
|
constructor(context: MigrationContext) {
|
|
this.context = context;
|
|
}
|
|
|
|
get db() {
|
|
return this.context.db;
|
|
}
|
|
|
|
get sequelize() {
|
|
return this.context.db.sequelize;
|
|
}
|
|
|
|
get queryInterface() {
|
|
return this.context.db.sequelize.getQueryInterface();
|
|
}
|
|
|
|
async up() {
|
|
// todo
|
|
}
|
|
|
|
async down() {
|
|
// todo
|
|
}
|
|
}
|
|
|
|
export interface MigrationItem {
|
|
name: string;
|
|
migration?: typeof Migration | string;
|
|
context?: any;
|
|
up?: any;
|
|
down?: any;
|
|
}
|
|
|
|
export class Migrations {
|
|
items = [];
|
|
context: any;
|
|
|
|
constructor(context: any) {
|
|
this.context = context;
|
|
}
|
|
|
|
clear() {
|
|
this.items = [];
|
|
}
|
|
|
|
add(item: MigrationItem) {
|
|
const Migration = item.migration;
|
|
|
|
if (Migration && typeof Migration === 'function') {
|
|
const migration = new Migration({ ...this.context, ...item.context });
|
|
migration.name = item.name;
|
|
this.items.push(migration);
|
|
} else {
|
|
this.items.push(item);
|
|
}
|
|
}
|
|
|
|
callback() {
|
|
return async (ctx) => {
|
|
return await Promise.all(
|
|
_.sortBy(this.items, (item) => {
|
|
const keys = item.name.split('/');
|
|
return keys.pop() || item.name;
|
|
}).map(async (item) => {
|
|
if (typeof item.migration === 'string') {
|
|
// use es module to import migration
|
|
const Migration = await importModule(item.migration);
|
|
const migration = new Migration({ ...this.context, ...item.context });
|
|
migration.name = item.name;
|
|
return migration;
|
|
}
|
|
|
|
return item;
|
|
}),
|
|
);
|
|
};
|
|
}
|
|
}
|