mirror of
https://github.com/nocobase/nocobase
synced 2024-11-16 00:46:53 +00:00
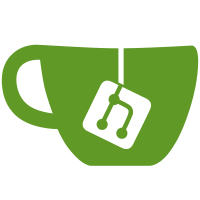
* create-nocobase-app template from [develop] * change create-nocobase-app package.json config * feat: load configuration from directory * feat: configuration repository toObject * feat: create application from configuration dir * feat: application factory with plugins options * export type * feat: read application config & application with plugins options * feat: release command * fix: database release * chore: workflow package.json * feat: nocobase cli package * feat: console command * chore: load application in command * fix: load packages from process.cwd * feat: cli load env file * feat: create-nocobase-app * fix: gitignore create-nocobase-app lib * fix: sqlite path * feat: create plugin * chore: plugin files template * chore: move cli into application * chore: create-nocobase-app * fix: create plugin * chore: app-client && app-server * chore: package.json * feat: create-nocobase-app download template from npm * chore: create-nocobase-app template * fix: config of plugin-users * fix: yarn.lock * fix: database build error * fix: yarn.lock * fix: resourcer config * chore: cross-env * chore: app-client dependents * fix: env * chore: v0.6.0-alpha.1 * chore: verdaccio * chore(versions): 😊 publish v0.6.0 * chore(versions): 😊 publish v0.6.1-alpha.0 * chore(versions): 😊 publish v0.6.2-alpha.0 * chore(versions): 😊 publish v0.6.2-alpha.1 * chore: 0.6.2-alpha.2 * feat: workspaces * chore(versions): 😊 publish v0.6.2-alpha.3 * chore(versions): 😊 publish v0.6.2-alpha.4 * chore: create-nocobase-app * chore: create-nocobase-app lib * fix: update tsconfig.jest.json * chore: .env * chore(versions): 😊 publish v0.6.2-alpha.5 * chore(versions): 😊 publish v0.6.2-alpha.6 * feat: improve code * chore(versions): 😊 publish v0.6.2-alpha.7 * fix: cleanup * chore(versions): 😊 publish v0.6.2-alpha.8 * chore: tsconfig for app server package * fix: move files * fix: move files Co-authored-by: chenos <chenlinxh@gmail.com>
122 lines
3.0 KiB
TypeScript
122 lines
3.0 KiB
TypeScript
import { ACL, DefineOptions } from './acl';
|
|
import { AvailableStrategyOptions } from './acl-available-strategy';
|
|
import { ACLResource } from './acl-resource';
|
|
|
|
export interface RoleActionParams {
|
|
fields?: string[];
|
|
filter?: any;
|
|
own?: boolean;
|
|
whitelist?: string[];
|
|
blacklist?: string[];
|
|
[key: string]: any;
|
|
}
|
|
|
|
interface ResourceActionsOptions {
|
|
[actionName: string]: RoleActionParams;
|
|
}
|
|
|
|
export class ACLRole {
|
|
strategy: string | AvailableStrategyOptions;
|
|
resources = new Map<string, ACLResource>();
|
|
|
|
constructor(public acl: ACL, public name: string) {}
|
|
|
|
getResource(name: string): ACLResource | undefined {
|
|
return this.resources.get(name);
|
|
}
|
|
|
|
setResource(name: string, resource: ACLResource) {
|
|
this.resources.set(name, resource);
|
|
}
|
|
|
|
public setStrategy(value: string | AvailableStrategyOptions) {
|
|
this.strategy = value;
|
|
}
|
|
|
|
public grantResource(resourceName: string, options: ResourceActionsOptions) {
|
|
const resource = new ACLResource({
|
|
role: this,
|
|
name: resourceName,
|
|
});
|
|
|
|
for (const [actionName, actionParams] of Object.entries(options)) {
|
|
resource.setAction(actionName, actionParams);
|
|
}
|
|
|
|
this.resources.set(resourceName, resource);
|
|
}
|
|
|
|
public getResourceActionsParams(resourceName: string) {
|
|
const resource = this.getResource(resourceName);
|
|
return resource.getActions();
|
|
}
|
|
|
|
public revokeResource(resourceName) {
|
|
for (const key of [...this.resources.keys()]) {
|
|
if (key === resourceName || key.includes(`${resourceName}.`)) {
|
|
this.resources.delete(key);
|
|
}
|
|
}
|
|
}
|
|
|
|
public grantAction(path: string, options?: RoleActionParams) {
|
|
let { resource, resourceName, actionName } = this.getResourceActionFromPath(path);
|
|
|
|
if (!resource) {
|
|
resource = new ACLResource({
|
|
role: this,
|
|
name: resourceName,
|
|
});
|
|
|
|
this.resources.set(resourceName, resource);
|
|
}
|
|
|
|
resource.setAction(actionName, options);
|
|
}
|
|
|
|
public getActionParams(path: string): RoleActionParams {
|
|
const { action } = this.getResourceActionFromPath(path);
|
|
return action;
|
|
}
|
|
|
|
public revokeAction(path: string) {
|
|
const { resource, actionName } = this.getResourceActionFromPath(path);
|
|
resource.removeAction(actionName);
|
|
}
|
|
|
|
public toJSON(): DefineOptions {
|
|
const actions = {};
|
|
|
|
for (const resourceName of this.resources.keys()) {
|
|
const resourceActions = this.getResourceActionsParams(resourceName);
|
|
for (const actionName of Object.keys(resourceActions)) {
|
|
actions[`${resourceName}:${actionName}`] = resourceActions[actionName];
|
|
}
|
|
}
|
|
|
|
return {
|
|
role: this.name,
|
|
strategy: this.strategy,
|
|
actions,
|
|
};
|
|
}
|
|
|
|
protected getResourceActionFromPath(path: string) {
|
|
const [resourceName, actionName] = path.split(':');
|
|
|
|
const resource = this.resources.get(resourceName);
|
|
|
|
let action = null;
|
|
if (resource) {
|
|
action = resource.getAction(actionName);
|
|
}
|
|
|
|
return {
|
|
resourceName,
|
|
actionName,
|
|
resource,
|
|
action,
|
|
};
|
|
}
|
|
}
|