mirror of
https://github.com/nocobase/nocobase
synced 2024-11-15 23:36:15 +00:00
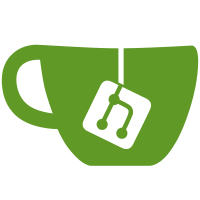
* feat: multiple apps plugin * feat: multipleAppManager in Application * stage * fix: export error * test: multiple app * application model * feat: create application with plugins * load and install after sub application created * create subApp database beforeInstall * sub apps listen to main app start & stop events * refactor: getPluginName as package name * feat: load apps on mainApp starts * fix: test * feat: beforeGetApplication event * fix: test * fix: test with sqlite memory database * test: lazyLoad application * fix: test with sqlite memory * chore: clone database collection & promise.all
70 lines
1.4 KiB
TypeScript
70 lines
1.4 KiB
TypeScript
import { Database } from '@nocobase/database';
|
|
import { Application } from './application';
|
|
import finder from 'find-package-json';
|
|
|
|
import { InstallOptions } from './plugin-manager';
|
|
|
|
export interface PluginInterface {
|
|
beforeLoad?: () => void;
|
|
load();
|
|
getName(): string;
|
|
}
|
|
|
|
export interface PluginOptions {
|
|
activate?: boolean;
|
|
displayName?: string;
|
|
description?: string;
|
|
version?: string;
|
|
install?: (this: Plugin) => void;
|
|
load?: (this: Plugin) => void;
|
|
plugin?: typeof Plugin;
|
|
[key: string]: any;
|
|
}
|
|
|
|
export type PluginType = typeof Plugin;
|
|
|
|
export abstract class Plugin<O = any> implements PluginInterface {
|
|
options: O;
|
|
app: Application;
|
|
db: Database;
|
|
|
|
constructor(app: Application, options?: O) {
|
|
this.app = app;
|
|
this.db = app.db;
|
|
this.setOptions(options);
|
|
}
|
|
|
|
setOptions(options: O) {
|
|
this.options = options || ({} as any);
|
|
}
|
|
|
|
getName(): string {
|
|
const path = require.main.children[require.main.children.length - 1].path;
|
|
|
|
return '';
|
|
}
|
|
|
|
beforeLoad() {}
|
|
|
|
async install(options?: InstallOptions) {}
|
|
|
|
async load() {
|
|
const collectionPath = this.collectionPath();
|
|
if (collectionPath) {
|
|
await this.db.import({
|
|
directory: collectionPath,
|
|
});
|
|
}
|
|
}
|
|
|
|
collectionPath() {
|
|
return null;
|
|
}
|
|
|
|
protected getPackageName(dirname: string) {
|
|
const f = finder(dirname);
|
|
const packageObj = f.next().value;
|
|
return packageObj['name'];
|
|
}
|
|
}
|