mirror of
https://github.com/occ-ai/obs-localvocal
synced 2024-11-08 03:08:07 +00:00
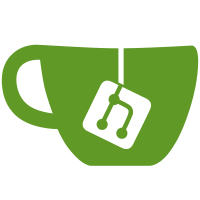
* refactor: Enable partial transcription with a latency of 1000ms * refactor: Update CMakePresets.json and buildspec.json - Remove the "QT_VERSION" variable from CMakePresets.json for all platforms - Update the "version" of "obs-studio" and "prebuilt" dependencies in buildspec.json - Update the "version" of "qt6" dependency in buildspec.json - Update the "version" of the project to "0.3.3" in buildspec.json - Update the "version" of the project to "0.3.3" in CMakePresets.json - Remove unused code in whisper-processing.cpp * refactor: Add -Wno-error=deprecated-declarations option to compilerconfig.cmake * refactor: Update language codes in translation module
53 lines
1.6 KiB
C++
53 lines
1.6 KiB
C++
#ifndef TRANSCRIPTION_UTILS_H
|
|
#define TRANSCRIPTION_UTILS_H
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
#include <chrono>
|
|
#include <algorithm>
|
|
#include <cctype>
|
|
|
|
// Fix UTF8 string for Windows
|
|
std::string fix_utf8(const std::string &str);
|
|
|
|
// Remove leading and trailing non-alphabetic characters
|
|
std::string remove_leading_trailing_nonalpha(const std::string &str);
|
|
|
|
// Split a string by a delimiter
|
|
std::vector<std::string> split(const std::string &string, char delimiter);
|
|
|
|
// Get the current timestamp in milliseconds since epoch
|
|
inline uint64_t now_ms()
|
|
{
|
|
return std::chrono::duration_cast<std::chrono::milliseconds>(
|
|
std::chrono::system_clock::now().time_since_epoch())
|
|
.count();
|
|
}
|
|
|
|
// Get the current timestamp in nano seconds since epoch
|
|
inline uint64_t now_ns()
|
|
{
|
|
return std::chrono::duration_cast<std::chrono::nanoseconds>(
|
|
std::chrono::system_clock::now().time_since_epoch())
|
|
.count();
|
|
}
|
|
|
|
// Split a string into words based on spaces
|
|
std::vector<std::string> split_words(const std::string &str_copy);
|
|
|
|
// trim (strip) string from leading and trailing whitespaces
|
|
template<typename StringLike> StringLike trim(const StringLike &str)
|
|
{
|
|
StringLike str_copy = str;
|
|
str_copy.erase(str_copy.begin(),
|
|
std::find_if(str_copy.begin(), str_copy.end(),
|
|
[](unsigned char ch) { return !std::isspace(ch); }));
|
|
str_copy.erase(std::find_if(str_copy.rbegin(), str_copy.rend(),
|
|
[](unsigned char ch) { return !std::isspace(ch); })
|
|
.base(),
|
|
str_copy.end());
|
|
return str_copy;
|
|
}
|
|
|
|
#endif // TRANSCRIPTION_UTILS_H
|