mirror of
https://github.com/OneUptime/oneuptime
synced 2024-11-22 15:24:55 +00:00
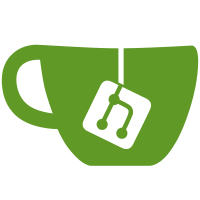
- Modify the Semaphore class in Semaphore.ts to include a namespace parameter in the lock method. - Update the lockTimeout default value from 1000 to 5000 milliseconds. - Append the namespace to the key when creating a new Mutex instance. - Add a try-catch block to handle errors when acquiring and releasing the mutex.
42 lines
861 B
TypeScript
42 lines
861 B
TypeScript
import Redis, { ClientType } from "./Redis";
|
|
import { Mutex } from "redis-semaphore";
|
|
|
|
export type SemaphoreMutex = Mutex;
|
|
|
|
export default class Semaphore {
|
|
// returns the mutex id
|
|
public static async lock(data: {
|
|
key: string;
|
|
namespace: string;
|
|
lockTimeout?: number;
|
|
}): Promise<SemaphoreMutex> {
|
|
if (!data.lockTimeout) {
|
|
data.lockTimeout = 5000;
|
|
}
|
|
|
|
const { key } = data;
|
|
|
|
const client: ClientType | null = Redis.getClient();
|
|
|
|
if (!client) {
|
|
throw new Error("Redis client is not connected");
|
|
}
|
|
|
|
const mutex: SemaphoreMutex = new Mutex(
|
|
client,
|
|
data.namespace + "-" + key,
|
|
{
|
|
lockTimeout: data.lockTimeout,
|
|
},
|
|
);
|
|
|
|
await mutex.acquire();
|
|
|
|
return mutex;
|
|
}
|
|
|
|
public static async release(mutex: SemaphoreMutex): Promise<void> {
|
|
await mutex.release();
|
|
}
|
|
}
|