mirror of
https://github.com/OneUptime/oneuptime
synced 2024-11-22 07:10:53 +00:00
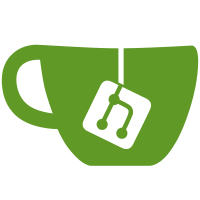
***Update GConstructor type in Mixins*** ***Update ReflectionMetadataType in Reflection*** ***Update connectToDatabase function in Redis*** ***Update MockRouterForMethodFunction in Helpers*** ***Update DefaultValidateFunction type in BasicForm*** ***Update result type in BaseAPI.test*** ***Update startUserNotificationRuleExecution function in OnCallDutyPolicyEscalationRuleService*** ***Update getTableColumn and getTableColumns functions in TableColumn*** ***Update getColumnBillingAccessControl and getColumnBillingAccessControlForAllColumns functions in ColumnBillingAccessControl
51 lines
1.6 KiB
TypeScript
51 lines
1.6 KiB
TypeScript
import fs from 'fs';
|
|
import { PromiseRejectErrorFunction } from 'Common/Types/FunctionTypes';
|
|
|
|
export default class LocalFile {
|
|
public static async makeDirectory(path: string): Promise<void> {
|
|
return new Promise(
|
|
(resolve: VoidFunction, reject: PromiseRejectErrorFunction) => {
|
|
fs.mkdir(path, { recursive: true }, (err: Error | null) => {
|
|
if (err) {
|
|
return reject(err);
|
|
}
|
|
resolve();
|
|
});
|
|
}
|
|
);
|
|
}
|
|
|
|
public static async write(path: string, data: string): Promise<void> {
|
|
return new Promise(
|
|
(resolve: VoidFunction, reject: PromiseRejectErrorFunction) => {
|
|
fs.writeFile(path, data, (err: Error | null) => {
|
|
if (err) {
|
|
return reject(err);
|
|
}
|
|
resolve();
|
|
});
|
|
}
|
|
);
|
|
}
|
|
|
|
public static async read(path: string): Promise<string> {
|
|
return new Promise(
|
|
(
|
|
resolve: (data: string) => void,
|
|
reject: PromiseRejectErrorFunction
|
|
) => {
|
|
fs.readFile(
|
|
path,
|
|
{ encoding: 'utf-8' },
|
|
(err: Error | null, data: string) => {
|
|
if (!err) {
|
|
return resolve(data);
|
|
}
|
|
return reject(err);
|
|
}
|
|
);
|
|
}
|
|
);
|
|
}
|
|
}
|