mirror of
https://github.com/OneUptime/oneuptime
synced 2024-11-22 07:10:53 +00:00
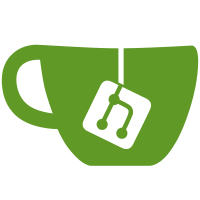
This commit updates the timeout handling in the monitor scripts to use the `WorkflowScriptTimeoutInMS` and `PROBE_CUSTOM_CODE_MONITOR_SCRIPT_TIMEOUT_IN_MS` values from the environment configuration. This ensures that the monitor scripts have consistent and configurable timeout values, improving the reliability and performance of the monitoring system.
78 lines
2.3 KiB
TypeScript
78 lines
2.3 KiB
TypeScript
import URL from 'Common/Types/API/URL';
|
|
import logger from 'CommonServer/Utils/Logger';
|
|
import ObjectID from 'Common/Types/ObjectID';
|
|
|
|
if (!process.env['INGESTOR_URL'] && !process.env['ONEUPTIME_URL']) {
|
|
logger.error('INGESTOR_URL or ONEUPTIME_URL is not set');
|
|
process.exit();
|
|
}
|
|
|
|
export let INGESTOR_URL: URL = URL.fromString(
|
|
process.env['ONEUPTIME_URL'] ||
|
|
process.env['INGESTOR_URL'] ||
|
|
'https://oneuptime.com'
|
|
);
|
|
|
|
// If probe api does not have the path. Add it.
|
|
if (
|
|
!INGESTOR_URL.toString().endsWith('ingestor') &&
|
|
!INGESTOR_URL.toString().endsWith('ingestor/')
|
|
) {
|
|
INGESTOR_URL = URL.fromString(
|
|
INGESTOR_URL.addRoute('/ingestor').toString()
|
|
);
|
|
}
|
|
|
|
export const PROBE_NAME: string | null = process.env['PROBE_NAME'] || null;
|
|
|
|
export const PROBE_DESCRIPTION: string | null =
|
|
process.env['PROBE_DESCRIPTION'] || null;
|
|
|
|
export const PROBE_ID: ObjectID | null = process.env['PROBE_ID']
|
|
? new ObjectID(process.env['PROBE_ID'])
|
|
: null;
|
|
|
|
if (!process.env['PROBE_KEY']) {
|
|
logger.error('PROBE_KEY is not set');
|
|
process.exit();
|
|
}
|
|
|
|
export const PROBE_KEY: string = process.env['PROBE_KEY'];
|
|
|
|
let probeMonitoringWorkers: string | number =
|
|
process.env['PROBE_MONITORING_WORKERS'] || 1;
|
|
|
|
if (typeof probeMonitoringWorkers === 'string') {
|
|
probeMonitoringWorkers = parseInt(probeMonitoringWorkers);
|
|
}
|
|
|
|
export const PROBE_MONITORING_WORKERS: number = probeMonitoringWorkers;
|
|
|
|
let monitorFetchLimit: string | number =
|
|
process.env['PROBE_MONITOR_FETCH_LIMIT'] || 1;
|
|
|
|
if (typeof monitorFetchLimit === 'string') {
|
|
monitorFetchLimit = parseInt(monitorFetchLimit);
|
|
}
|
|
|
|
export const PROBE_MONITOR_FETCH_LIMIT: number = monitorFetchLimit;
|
|
|
|
export const HOSTNAME: string = process.env['HOSTNAME'] || 'localhost';
|
|
|
|
export const PROBE_SYNTHETIC_MONITOR_SCRIPT_TIMEOUT_IN_MS: number = process.env[
|
|
'PROBE_SYNTHETIC_MONITOR_SCRIPT_TIMEOUT_IN_MS'
|
|
]
|
|
? parseInt(
|
|
process.env['PROBE_SYNTHETIC_MONITOR_SCRIPT_TIMEOUT_IN_MS'].toString()
|
|
)
|
|
: 60000;
|
|
|
|
export const PROBE_CUSTOM_CODE_MONITOR_SCRIPT_TIMEOUT_IN_MS: number = process
|
|
.env['PROBE_CUSTOM_CODE_MONITOR_SCRIPT_TIMEOUT_IN_MS']
|
|
? parseInt(
|
|
process.env[
|
|
'PROBE_CUSTOM_CODE_MONITOR_SCRIPT_TIMEOUT_IN_MS'
|
|
].toString()
|
|
)
|
|
: 60000;
|