mirror of
http://github.com/valkey-io/valkey
synced 2024-11-21 16:46:15 +00:00
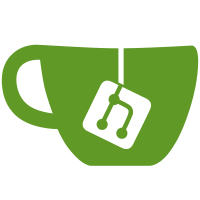
The core idea was to take a lot of the stuff from the C unity framework and adapt it a bit here. Each file in the `unit` directory that starts with `test_` is automatically assumed to be a test suite. Within each file, all functions that start with `test_` are assumed to be a test. See unit/README.md for details about the implementation. Instead of compiling basically a net new binary, the way the tests are compiled is that the main valkey server is compiled as a static archive, which we then compile the individual test files against to create a new test executable. This is not all that important now, other than it makes the compilation simpler, but what it will allow us to do is overwrite functions in the archive to enable mocking for cross compilation unit functions. There are also ways to enable mocking from within the same compilation unit, but I don't know how important this is. Tests are also written in one of two styles: 1. Including the header file and directly calling functions from the archive. 2. Importing the original file, and then calling the functions. This second approach is cool because we can call static functions. It won't mess up the archive either. --------- Signed-off-by: Madelyn Olson <madelyneolson@gmail.com>
59 lines
2.2 KiB
Python
Executable File
59 lines
2.2 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
import os
|
|
import re
|
|
|
|
UNIT_DIR = os.path.abspath(os.path.dirname(os.path.abspath(__file__)) + '/../src/unit')
|
|
TEST_FILE = UNIT_DIR + '/test_files.h'
|
|
TEST_PROTOTYPE = '(int (test_[a-zA-Z0-9_]*)\(.*\)).*{'
|
|
|
|
if __name__ == '__main__':
|
|
with open(TEST_FILE, 'w') as output:
|
|
# Find each test file and collect the test names.
|
|
test_suites = []
|
|
for root, dirs, files in os.walk(UNIT_DIR):
|
|
for file in files:
|
|
file_path = UNIT_DIR + '/' + file
|
|
if not file.endswith('.c') or file == 'test_main.c':
|
|
continue
|
|
tests = []
|
|
with open(file_path, 'r') as f:
|
|
for line in f:
|
|
match = re.match(TEST_PROTOTYPE, line)
|
|
if match:
|
|
function = match.group(1)
|
|
test_name = match.group(2)
|
|
tests.append((test_name, function))
|
|
test_suites.append({'file': file, 'tests': tests})
|
|
test_suites.sort(key=lambda test_suite: test_suite['file'])
|
|
output.write("""/* Do not modify this file, it's automatically generated from utils/generate-unit-test-header.py */
|
|
typedef int unitTestProc(int argc, char **argv, int flags);
|
|
|
|
typedef struct unitTest {
|
|
char *name;
|
|
unitTestProc *proc;
|
|
} unitTest;
|
|
|
|
""")
|
|
|
|
# Write the headers for the functions
|
|
for test_suite in test_suites:
|
|
for test in test_suite['tests']:
|
|
output.write('{};\n'.format(test[1]))
|
|
output.write("\n")
|
|
|
|
# Create test suite lists
|
|
for test_suite in test_suites:
|
|
output.write('unitTest __{}[] = {{'.format(test_suite['file'].replace('.c', '_c')))
|
|
for test in test_suite['tests']:
|
|
output.write('{{"{}", {}}}, '.format(test[0], test[0]))
|
|
output.write('{NULL, NULL}};\n')
|
|
|
|
output.write("""
|
|
struct unitTestSuite {
|
|
char *filename;
|
|
unitTest *tests;
|
|
} unitTestSuite[] = {
|
|
""")
|
|
for test_suite in test_suites:
|
|
output.write(' {{"{0}", __{1}}},\n'.format(test_suite['file'], test_suite['file'].replace('.c', '_c')))
|
|
output.write('};\n') |