mirror of
http://github.com/valkey-io/valkey
synced 2024-11-22 09:17:20 +00:00
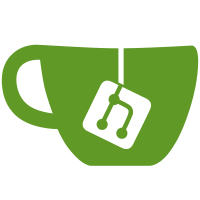
This allows modules to register commands to existing ACL categories and blocks the creation of [sub]commands, datatypes and registering the configs outside of the OnLoad function. For allowing modules to register commands to existing ACL categories, This PR implements a new API int RM_SetCommandACLCategories() which takes a pointer to a RedisModuleCommand and a C string aclflags containing the set of space separated ACL categories. Example, 'write slow' marks the command as part of the write and slow ACL categories. The C string aclflags is tokenized by implementing a helper function categoryFlagsFromString(). Theses tokens are matched and the corresponding ACL categories flags are set by a helper function matchAclCategoriesFlags. The helper function categoryFlagsFromString() returns the corresponding categories_flags or returns -1 if some token not processed correctly. If the module contains commands which are registered to existing ACL categories, the number of [sub]commands are tracked by num_commands_with_acl_categories in struct RedisModule. Further, the allowed command bit-map of the existing users are recomputed from the command_rules list, by implementing a function called ACLRecomputeCommandBitsFromCommandRulesAllUsers() for the existing users to have access to the module commands on runtime. ## Breaking change This change requires that registering commands and subcommands only occur during a modules "OnLoad" function, in order to allow efficient recompilation of ACL bits. We also chose to block registering configs and types, since we believe it's only valid for those to be created during onLoad. We check for this onload flag in struct RedisModule to check if the call is made from the OnLoad function. Co-authored-by: Madelyn Olson <madelyneolson@gmail.com>
196 lines
7.8 KiB
C
196 lines
7.8 KiB
C
#include "redismodule.h"
|
|
#include <strings.h>
|
|
int mutable_bool_val;
|
|
int immutable_bool_val;
|
|
long long longval;
|
|
long long memval;
|
|
RedisModuleString *strval = NULL;
|
|
int enumval;
|
|
int flagsval;
|
|
|
|
/* Series of get and set callbacks for each type of config, these rely on the privdata ptr
|
|
* to point to the config, and they register the configs as such. Note that one could also just
|
|
* use names if they wanted, and store anything in privdata. */
|
|
int getBoolConfigCommand(const char *name, void *privdata) {
|
|
REDISMODULE_NOT_USED(name);
|
|
return (*(int *)privdata);
|
|
}
|
|
|
|
int setBoolConfigCommand(const char *name, int new, void *privdata, RedisModuleString **err) {
|
|
REDISMODULE_NOT_USED(name);
|
|
REDISMODULE_NOT_USED(err);
|
|
*(int *)privdata = new;
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
long long getNumericConfigCommand(const char *name, void *privdata) {
|
|
REDISMODULE_NOT_USED(name);
|
|
return (*(long long *) privdata);
|
|
}
|
|
|
|
int setNumericConfigCommand(const char *name, long long new, void *privdata, RedisModuleString **err) {
|
|
REDISMODULE_NOT_USED(name);
|
|
REDISMODULE_NOT_USED(err);
|
|
*(long long *)privdata = new;
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
RedisModuleString *getStringConfigCommand(const char *name, void *privdata) {
|
|
REDISMODULE_NOT_USED(name);
|
|
REDISMODULE_NOT_USED(privdata);
|
|
return strval;
|
|
}
|
|
int setStringConfigCommand(const char *name, RedisModuleString *new, void *privdata, RedisModuleString **err) {
|
|
REDISMODULE_NOT_USED(name);
|
|
REDISMODULE_NOT_USED(err);
|
|
REDISMODULE_NOT_USED(privdata);
|
|
size_t len;
|
|
if (!strcasecmp(RedisModule_StringPtrLen(new, &len), "rejectisfreed")) {
|
|
*err = RedisModule_CreateString(NULL, "Cannot set string to 'rejectisfreed'", 36);
|
|
return REDISMODULE_ERR;
|
|
}
|
|
if (strval) RedisModule_FreeString(NULL, strval);
|
|
RedisModule_RetainString(NULL, new);
|
|
strval = new;
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
int getEnumConfigCommand(const char *name, void *privdata) {
|
|
REDISMODULE_NOT_USED(name);
|
|
REDISMODULE_NOT_USED(privdata);
|
|
return enumval;
|
|
}
|
|
|
|
int setEnumConfigCommand(const char *name, int val, void *privdata, RedisModuleString **err) {
|
|
REDISMODULE_NOT_USED(name);
|
|
REDISMODULE_NOT_USED(err);
|
|
REDISMODULE_NOT_USED(privdata);
|
|
enumval = val;
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
int getFlagsConfigCommand(const char *name, void *privdata) {
|
|
REDISMODULE_NOT_USED(name);
|
|
REDISMODULE_NOT_USED(privdata);
|
|
return flagsval;
|
|
}
|
|
|
|
int setFlagsConfigCommand(const char *name, int val, void *privdata, RedisModuleString **err) {
|
|
REDISMODULE_NOT_USED(name);
|
|
REDISMODULE_NOT_USED(err);
|
|
REDISMODULE_NOT_USED(privdata);
|
|
flagsval = val;
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
int boolApplyFunc(RedisModuleCtx *ctx, void *privdata, RedisModuleString **err) {
|
|
REDISMODULE_NOT_USED(ctx);
|
|
REDISMODULE_NOT_USED(privdata);
|
|
if (mutable_bool_val && immutable_bool_val) {
|
|
*err = RedisModule_CreateString(NULL, "Bool configs cannot both be yes.", 32);
|
|
return REDISMODULE_ERR;
|
|
}
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
int longlongApplyFunc(RedisModuleCtx *ctx, void *privdata, RedisModuleString **err) {
|
|
REDISMODULE_NOT_USED(ctx);
|
|
REDISMODULE_NOT_USED(privdata);
|
|
if (longval == memval) {
|
|
*err = RedisModule_CreateString(NULL, "These configs cannot equal each other.", 38);
|
|
return REDISMODULE_ERR;
|
|
}
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
int registerBlockCheck(RedisModuleCtx *ctx, RedisModuleString **argv, int argc) {
|
|
REDISMODULE_NOT_USED(argv);
|
|
REDISMODULE_NOT_USED(argc);
|
|
int response_ok = 0;
|
|
int result = RedisModule_RegisterBoolConfig(ctx, "mutable_bool", 1, REDISMODULE_CONFIG_DEFAULT, getBoolConfigCommand, setBoolConfigCommand, boolApplyFunc, &mutable_bool_val);
|
|
response_ok |= (result == REDISMODULE_OK);
|
|
|
|
result = RedisModule_RegisterStringConfig(ctx, "string", "secret password", REDISMODULE_CONFIG_DEFAULT, getStringConfigCommand, setStringConfigCommand, NULL, NULL);
|
|
response_ok |= (result == REDISMODULE_OK);
|
|
|
|
const char *enum_vals[] = {"none", "five", "one", "two", "four"};
|
|
const int int_vals[] = {0, 5, 1, 2, 4};
|
|
result = RedisModule_RegisterEnumConfig(ctx, "enum", 1, REDISMODULE_CONFIG_DEFAULT, enum_vals, int_vals, 5, getEnumConfigCommand, setEnumConfigCommand, NULL, NULL);
|
|
response_ok |= (result == REDISMODULE_OK);
|
|
|
|
result = RedisModule_RegisterNumericConfig(ctx, "numeric", -1, REDISMODULE_CONFIG_DEFAULT, -5, 2000, getNumericConfigCommand, setNumericConfigCommand, longlongApplyFunc, &longval);
|
|
response_ok |= (result == REDISMODULE_OK);
|
|
|
|
result = RedisModule_LoadConfigs(ctx);
|
|
response_ok |= (result == REDISMODULE_OK);
|
|
|
|
/* This validates that it's not possible to register/load configs outside OnLoad,
|
|
* thus returns an error if they succeed. */
|
|
if (response_ok) {
|
|
RedisModule_ReplyWithError(ctx, "UNEXPECTEDOK");
|
|
} else {
|
|
RedisModule_ReplyWithSimpleString(ctx, "OK");
|
|
}
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
int RedisModule_OnLoad(RedisModuleCtx *ctx, RedisModuleString **argv, int argc) {
|
|
REDISMODULE_NOT_USED(argv);
|
|
REDISMODULE_NOT_USED(argc);
|
|
|
|
if (RedisModule_Init(ctx, "moduleconfigs", 1, REDISMODULE_APIVER_1) == REDISMODULE_ERR) return REDISMODULE_ERR;
|
|
|
|
if (RedisModule_RegisterBoolConfig(ctx, "mutable_bool", 1, REDISMODULE_CONFIG_DEFAULT, getBoolConfigCommand, setBoolConfigCommand, boolApplyFunc, &mutable_bool_val) == REDISMODULE_ERR) {
|
|
return REDISMODULE_ERR;
|
|
}
|
|
/* Immutable config here. */
|
|
if (RedisModule_RegisterBoolConfig(ctx, "immutable_bool", 0, REDISMODULE_CONFIG_IMMUTABLE, getBoolConfigCommand, setBoolConfigCommand, boolApplyFunc, &immutable_bool_val) == REDISMODULE_ERR) {
|
|
return REDISMODULE_ERR;
|
|
}
|
|
if (RedisModule_RegisterStringConfig(ctx, "string", "secret password", REDISMODULE_CONFIG_DEFAULT, getStringConfigCommand, setStringConfigCommand, NULL, NULL) == REDISMODULE_ERR) {
|
|
return REDISMODULE_ERR;
|
|
}
|
|
|
|
/* On the stack to make sure we're copying them. */
|
|
const char *enum_vals[] = {"none", "five", "one", "two", "four"};
|
|
const int int_vals[] = {0, 5, 1, 2, 4};
|
|
|
|
if (RedisModule_RegisterEnumConfig(ctx, "enum", 1, REDISMODULE_CONFIG_DEFAULT, enum_vals, int_vals, 5, getEnumConfigCommand, setEnumConfigCommand, NULL, NULL) == REDISMODULE_ERR) {
|
|
return REDISMODULE_ERR;
|
|
}
|
|
if (RedisModule_RegisterEnumConfig(ctx, "flags", 3, REDISMODULE_CONFIG_DEFAULT | REDISMODULE_CONFIG_BITFLAGS, enum_vals, int_vals, 5, getFlagsConfigCommand, setFlagsConfigCommand, NULL, NULL) == REDISMODULE_ERR) {
|
|
return REDISMODULE_ERR;
|
|
}
|
|
/* Memory config here. */
|
|
if (RedisModule_RegisterNumericConfig(ctx, "memory_numeric", 1024, REDISMODULE_CONFIG_DEFAULT | REDISMODULE_CONFIG_MEMORY, 0, 3000000, getNumericConfigCommand, setNumericConfigCommand, longlongApplyFunc, &memval) == REDISMODULE_ERR) {
|
|
return REDISMODULE_ERR;
|
|
}
|
|
if (RedisModule_RegisterNumericConfig(ctx, "numeric", -1, REDISMODULE_CONFIG_DEFAULT, -5, 2000, getNumericConfigCommand, setNumericConfigCommand, longlongApplyFunc, &longval) == REDISMODULE_ERR) {
|
|
return REDISMODULE_ERR;
|
|
}
|
|
size_t len;
|
|
if (argc && !strcasecmp(RedisModule_StringPtrLen(argv[0], &len), "noload")) {
|
|
return REDISMODULE_OK;
|
|
} else if (RedisModule_LoadConfigs(ctx) == REDISMODULE_ERR) {
|
|
if (strval) {
|
|
RedisModule_FreeString(ctx, strval);
|
|
strval = NULL;
|
|
}
|
|
return REDISMODULE_ERR;
|
|
}
|
|
/* Creates a command which registers configs outside OnLoad() function. */
|
|
if (RedisModule_CreateCommand(ctx,"block.register.configs.outside.onload", registerBlockCheck, "write", 0, 0, 0) == REDISMODULE_ERR)
|
|
return REDISMODULE_ERR;
|
|
|
|
return REDISMODULE_OK;
|
|
}
|
|
|
|
int RedisModule_OnUnload(RedisModuleCtx *ctx) {
|
|
REDISMODULE_NOT_USED(ctx);
|
|
if (strval) {
|
|
RedisModule_FreeString(ctx, strval);
|
|
strval = NULL;
|
|
}
|
|
return REDISMODULE_OK;
|
|
}
|