mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 18:44:40 +00:00
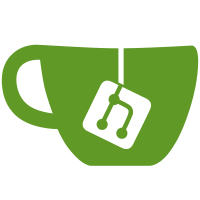
* project quota added * project quota removed * add periods table * make log record generic * accumulate usage * query usage * count action run seconds * fix filter in ReportQuotaUsage * fix existing tests * fix logstore tests * fix typo * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * move notifications into debouncer and improve limit querying * cleanup * comment * fix: add quota unit tests command side * fix remaining quota usage query * implement InmemLogStorage * cleanup and linting * improve test * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * fix: add quota unit tests command side * action notifications and fixes for notifications query * revert console prefix * fix: add quota unit tests command side * fix: add quota integration tests * improve accountable requests * improve accountable requests * fix: add quota integration tests * fix: add quota integration tests * fix: add quota integration tests * comment * remove ability to store logs in db and other changes requested from review * changes requested from review * changes requested from review * Update internal/api/http/middleware/access_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * tests: fix quotas integration tests * improve incrementUsageStatement * linting * fix: delete e2e tests as intergation tests cover functionality * Update internal/api/http/middleware/access_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * backup * fix conflict * create rc * create prerelease * remove issue release labeling * fix tracing --------- Co-authored-by: Livio Spring <livio.a@gmail.com> Co-authored-by: Stefan Benz <stefan@caos.ch> Co-authored-by: adlerhurst <silvan.reusser@gmail.com>
75 lines
1.4 KiB
Go
75 lines
1.4 KiB
Go
package logstore_test
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/logstore"
|
|
"github.com/zitadel/zitadel/internal/query"
|
|
)
|
|
|
|
type emitterOption func(config *logstore.EmitterConfig)
|
|
|
|
func emitterConfig(options ...emitterOption) *logstore.EmitterConfig {
|
|
cfg := &logstore.EmitterConfig{
|
|
Enabled: true,
|
|
Debounce: &logstore.DebouncerConfig{
|
|
MinFrequency: 0,
|
|
MaxBulkSize: 0,
|
|
},
|
|
}
|
|
for _, opt := range options {
|
|
opt(cfg)
|
|
}
|
|
return cfg
|
|
}
|
|
|
|
func withDebouncerConfig(config *logstore.DebouncerConfig) emitterOption {
|
|
return func(c *logstore.EmitterConfig) {
|
|
c.Debounce = config
|
|
}
|
|
}
|
|
|
|
func withDisabled() emitterOption {
|
|
return func(c *logstore.EmitterConfig) {
|
|
c.Enabled = false
|
|
}
|
|
}
|
|
|
|
type quotaOption func(config *query.Quota)
|
|
|
|
func quotaConfig(quotaOptions ...quotaOption) *query.Quota {
|
|
q := &query.Quota{
|
|
Amount: 90,
|
|
Limit: false,
|
|
ResetInterval: 90 * time.Second,
|
|
From: time.Unix(0, 0),
|
|
}
|
|
for _, opt := range quotaOptions {
|
|
opt(q)
|
|
}
|
|
return q
|
|
}
|
|
|
|
func withAmountAndInterval(n uint64) quotaOption {
|
|
return func(c *query.Quota) {
|
|
c.Amount = n
|
|
c.ResetInterval = time.Duration(n) * time.Second
|
|
}
|
|
}
|
|
|
|
func withLimiting() quotaOption {
|
|
return func(c *query.Quota) {
|
|
c.Limit = true
|
|
}
|
|
}
|
|
|
|
func repeat(value, times int) []int {
|
|
ints := make([]int, times)
|
|
for i := 0; i < times; i++ {
|
|
ints[i] = value
|
|
}
|
|
return ints
|
|
}
|
|
|
|
func uint64Ptr(n uint64) *uint64 { return &n }
|