mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 18:44:40 +00:00
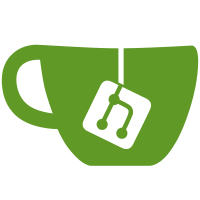
* feat: add execution of targets to grpc calls * feat: add execution of targets to grpc calls * feat: add execution of targets to grpc calls * feat: add execution of targets to grpc calls * feat: add execution of targets to grpc calls * feat: add execution of targets to grpc calls * feat: add execution of targets to grpc calls * feat: split request and response logic to handle the different context information * feat: split request and response logic to handle the different context information * fix: integration test * fix: import alias * fix: refactor execution package * fix: refactor execution interceptor integration and unit tests * fix: refactor execution interceptor integration and unit tests * fix: refactor execution interceptor integration and unit tests * fix: refactor execution interceptor integration and unit tests * fix: refactor execution interceptor integration and unit tests * docs: basic documentation for executions and targets * fix: change order for interceptors * fix: merge back origin/main * fix: change target definition command and query side (#7735) * fix: change target definition command and query side * fix: correct refactoring name changes * fix: correct refactoring name changes * fix: changing execution defintion with target list and type * fix: changing execution definition with target list and type * fix: add back search queries for target and include * fix: projections change for execution with targets suffix table * fix: projections change for execution with targets suffix table * fix: projections change for execution with targets suffix table * fix: projections change for execution with targets suffix table * fix: projections change for execution with targets suffix table * fix: projections change for execution with targets suffix table * fix: projections change for execution with targets suffix table * docs: add example to actions v2 * docs: add example to actions v2 * fix: correct integration tests on query for executions * fix: add separate event for execution v2 as content changed * fix: add separate event for execution v2 as content changed * fix: added review comment changes * fix: added review comment changes * fix: added review comment changes --------- Co-authored-by: adlerhurst <silvan.reusser@gmail.com> * fix: added review comment changes * fix: added review comment changes * Update internal/api/grpc/server/middleware/execution_interceptor.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * fix: added review comment changes * fix: added review comment changes * fix: added review comment changes * fix: added review comment changes * fix: added review comment changes * fix: added review comment changes --------- Co-authored-by: adlerhurst <silvan.reusser@gmail.com> Co-authored-by: Elio Bischof <elio@zitadel.com>
74 lines
2.2 KiB
Go
74 lines
2.2 KiB
Go
package integration
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
"google.golang.org/protobuf/types/known/timestamppb"
|
|
|
|
object "github.com/zitadel/zitadel/pkg/grpc/object/v2beta"
|
|
)
|
|
|
|
// Details is the interface that covers both v1 and v2 proto generated object details.
|
|
type Details interface {
|
|
comparable
|
|
GetSequence() uint64
|
|
GetChangeDate() *timestamppb.Timestamp
|
|
GetResourceOwner() string
|
|
}
|
|
|
|
// DetailsMsg is the interface that covers all proto messages which contain v1 or v2 object details.
|
|
type DetailsMsg[D Details] interface {
|
|
GetDetails() D
|
|
}
|
|
|
|
type ListDetailsMsg interface {
|
|
GetDetails() *object.ListDetails
|
|
}
|
|
|
|
// AssertDetails asserts values in a message's object Details,
|
|
// if the object Details in expected is a non-nil value.
|
|
// It targets API v2 messages that have the `GetDetails()` method.
|
|
//
|
|
// Dynamically generated values are not compared with expected.
|
|
// Instead a sanity check is performed.
|
|
// For the sequence a non-zero value is expected.
|
|
// If the change date is populated, it is checked with a tolerance of 1 minute around Now.
|
|
//
|
|
// The resource owner is compared with expected.
|
|
func AssertDetails[D Details, M DetailsMsg[D]](t testing.TB, expected, actual M) {
|
|
wantDetails, gotDetails := expected.GetDetails(), actual.GetDetails()
|
|
var nilDetails D
|
|
if wantDetails == nilDetails {
|
|
assert.Nil(t, gotDetails)
|
|
return
|
|
}
|
|
|
|
assert.NotZero(t, gotDetails.GetSequence())
|
|
|
|
if wantDetails.GetChangeDate() != nil {
|
|
wantChangeDate := time.Now()
|
|
gotChangeDate := gotDetails.GetChangeDate().AsTime()
|
|
assert.WithinRange(t, gotChangeDate, wantChangeDate.Add(-time.Minute), wantChangeDate.Add(time.Minute))
|
|
}
|
|
|
|
assert.Equal(t, wantDetails.GetResourceOwner(), gotDetails.GetResourceOwner())
|
|
}
|
|
|
|
func AssertListDetails[D ListDetailsMsg](t testing.TB, expected, actual D) {
|
|
wantDetails, gotDetails := expected.GetDetails(), actual.GetDetails()
|
|
if wantDetails == nil {
|
|
assert.Nil(t, gotDetails)
|
|
return
|
|
}
|
|
|
|
assert.Equal(t, wantDetails.GetTotalResult(), gotDetails.GetTotalResult())
|
|
|
|
if wantDetails.GetTimestamp() != nil {
|
|
gotCD := gotDetails.GetTimestamp().AsTime()
|
|
wantCD := time.Now()
|
|
assert.WithinRange(t, gotCD, wantCD.Add(-time.Minute), wantCD.Add(time.Minute))
|
|
}
|
|
}
|