mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 08:49:13 +00:00
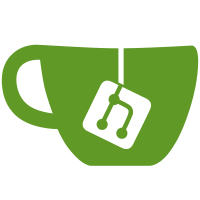
* partial work done * test IAM membership roles * org membership tests * console :(, translations and docs * fix integration test * fix tests * add EnableImpersonation to security policy API * fix integration test timestamp checking * add security policy tests and fix projections * add impersonation setting in console * add security settings to the settings v2 API * fix typo * move impersonation to instance --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
53 lines
902 B
Go
53 lines
902 B
Go
package integration
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"google.golang.org/protobuf/types/known/timestamppb"
|
|
|
|
object "github.com/zitadel/zitadel/pkg/grpc/object/v2beta"
|
|
)
|
|
|
|
type myMsg struct {
|
|
details *object.Details
|
|
}
|
|
|
|
func (m myMsg) GetDetails() *object.Details {
|
|
return m.details
|
|
}
|
|
|
|
func TestAssertDetails(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
exptected myMsg
|
|
actual myMsg
|
|
}{
|
|
{
|
|
name: "nil",
|
|
exptected: myMsg{},
|
|
actual: myMsg{},
|
|
},
|
|
{
|
|
name: "values",
|
|
exptected: myMsg{
|
|
details: &object.Details{
|
|
ResourceOwner: "me",
|
|
ChangeDate: timestamppb.Now(),
|
|
},
|
|
},
|
|
actual: myMsg{
|
|
details: &object.Details{
|
|
Sequence: 123,
|
|
ChangeDate: timestamppb.Now(),
|
|
ResourceOwner: "me",
|
|
},
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
AssertDetails(t, tt.exptected, tt.actual)
|
|
})
|
|
}
|
|
}
|