mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
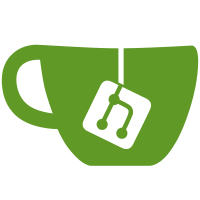
This implementation increases parallel write capabilities of the eventstore. Please have a look at the technical advisories: [05](https://zitadel.com/docs/support/advisory/a10005) and [06](https://zitadel.com/docs/support/advisory/a10006). The implementation of eventstore.push is rewritten and stored events are migrated to a new table `eventstore.events2`. If you are using cockroach: make sure that the database user of ZITADEL has `VIEWACTIVITY` grant. This is used to query events.
81 lines
1.9 KiB
Go
81 lines
1.9 KiB
Go
package eventstore
|
|
|
|
type UniqueConstraint struct {
|
|
// UniqueType is the table name for the unique constraint
|
|
UniqueType string
|
|
// UniqueField is the unique key
|
|
UniqueField string
|
|
// Action defines if unique constraint should be added or removed
|
|
Action UniqueConstraintAction
|
|
// ErrorMessage defines the translation file key for the error message
|
|
ErrorMessage string
|
|
// IsGlobal defines if the unique constraint is globally unique or just within a single instance
|
|
IsGlobal bool
|
|
}
|
|
|
|
type UniqueConstraintAction int8
|
|
|
|
const (
|
|
UniqueConstraintAdd UniqueConstraintAction = iota
|
|
UniqueConstraintRemove
|
|
UniqueConstraintInstanceRemove
|
|
|
|
uniqueConstraintActionCount
|
|
)
|
|
|
|
func (f UniqueConstraintAction) Valid() bool {
|
|
return f >= 0 && f < uniqueConstraintActionCount
|
|
}
|
|
|
|
func NewAddEventUniqueConstraint(
|
|
uniqueType,
|
|
uniqueField,
|
|
errMessage string) *UniqueConstraint {
|
|
return &UniqueConstraint{
|
|
UniqueType: uniqueType,
|
|
UniqueField: uniqueField,
|
|
ErrorMessage: errMessage,
|
|
Action: UniqueConstraintAdd,
|
|
}
|
|
}
|
|
|
|
func NewRemoveUniqueConstraint(
|
|
uniqueType,
|
|
uniqueField string) *UniqueConstraint {
|
|
return &UniqueConstraint{
|
|
UniqueType: uniqueType,
|
|
UniqueField: uniqueField,
|
|
Action: UniqueConstraintRemove,
|
|
}
|
|
}
|
|
|
|
func NewRemoveInstanceUniqueConstraints() *UniqueConstraint {
|
|
return &UniqueConstraint{
|
|
Action: UniqueConstraintInstanceRemove,
|
|
}
|
|
}
|
|
|
|
func NewAddGlobalUniqueConstraint(
|
|
uniqueType,
|
|
uniqueField,
|
|
errMessage string) *UniqueConstraint {
|
|
return &UniqueConstraint{
|
|
UniqueType: uniqueType,
|
|
UniqueField: uniqueField,
|
|
ErrorMessage: errMessage,
|
|
IsGlobal: true,
|
|
Action: UniqueConstraintAdd,
|
|
}
|
|
}
|
|
|
|
func NewRemoveGlobalUniqueConstraint(
|
|
uniqueType,
|
|
uniqueField string) *UniqueConstraint {
|
|
return &UniqueConstraint{
|
|
UniqueType: uniqueType,
|
|
UniqueField: uniqueField,
|
|
IsGlobal: true,
|
|
Action: UniqueConstraintRemove,
|
|
}
|
|
}
|