mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
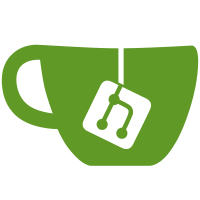
chore(fmt): run gci on complete project Fix global import formatting in go code by running the `gci` command. This allows us to just use the command directly, instead of fixing the import order manually for the linter, on each PR. Co-authored-by: Elio Bischof <elio@zitadel.com>
65 lines
1.6 KiB
Go
65 lines
1.6 KiB
Go
package query
|
|
|
|
import (
|
|
"time"
|
|
|
|
sq "github.com/Masterminds/squirrel"
|
|
|
|
"github.com/zitadel/zitadel/internal/database"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
)
|
|
|
|
type MembersQuery struct {
|
|
SearchRequest
|
|
Queries []SearchQuery
|
|
}
|
|
|
|
func (q *MembersQuery) toQuery(query sq.SelectBuilder) sq.SelectBuilder {
|
|
query = q.SearchRequest.toQuery(query)
|
|
for _, q := range q.Queries {
|
|
query = q.toQuery(query)
|
|
}
|
|
return query
|
|
}
|
|
|
|
func NewMemberEmailSearchQuery(method TextComparison, value string) (SearchQuery, error) {
|
|
return NewTextQuery(HumanEmailCol, value, method)
|
|
}
|
|
|
|
func NewMemberFirstNameSearchQuery(method TextComparison, value string) (SearchQuery, error) {
|
|
return NewTextQuery(HumanFirstNameCol, value, method)
|
|
}
|
|
|
|
func NewMemberLastNameSearchQuery(method TextComparison, value string) (SearchQuery, error) {
|
|
return NewTextQuery(HumanLastNameCol, value, method)
|
|
}
|
|
|
|
func NewMemberUserIDSearchQuery(value string) (SearchQuery, error) {
|
|
return NewTextQuery(membershipUserID, value, TextEquals)
|
|
}
|
|
func NewMemberResourceOwnerSearchQuery(value string) (SearchQuery, error) {
|
|
return NewTextQuery(membershipResourceOwner, value, TextEquals)
|
|
}
|
|
|
|
type Members struct {
|
|
SearchResponse
|
|
Members []*Member
|
|
}
|
|
|
|
type Member struct {
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
Sequence uint64
|
|
ResourceOwner string
|
|
|
|
UserID string
|
|
Roles database.TextArray[string]
|
|
PreferredLoginName string
|
|
Email string
|
|
FirstName string
|
|
LastName string
|
|
DisplayName string
|
|
AvatarURL string
|
|
UserType domain.UserType
|
|
}
|