mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 18:44:40 +00:00
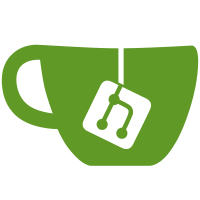
* docs: simplify traefik external tls * remove pass host header * docs: simplify and fix nginx external tls * fix: readiness with enabled tls * improve proxy docs * improve proxy docs * fix(ready): don't verify server cert * complete nginx docs * cleanup * complete traefik docs * add caddy docs * simplify traefik * standardize * fix caddy * add httpd docs * improve external config docs * guiding error message * docs(defaults.yaml): remove misleading comments * guiding error message cs and ru * improve proxy testability * fix compose up command * improve commands * fix nginx tls disabled * fix nginx tls enabled * fix: serve gateway when tls is enabled * fmt caddy files * fix caddy enabled tls * remove not-working commands * review * fix checks * fix link --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
48 lines
1.1 KiB
Go
48 lines
1.1 KiB
Go
package ready
|
|
|
|
import (
|
|
"crypto/tls"
|
|
"net"
|
|
"net/http"
|
|
"os"
|
|
"strconv"
|
|
|
|
"github.com/spf13/cobra"
|
|
"github.com/spf13/viper"
|
|
"github.com/zitadel/logging"
|
|
)
|
|
|
|
func New() *cobra.Command {
|
|
return &cobra.Command{
|
|
Use: "ready",
|
|
Short: "Checks if zitadel is ready",
|
|
Long: "Checks if zitadel is ready",
|
|
Run: func(cmd *cobra.Command, args []string) {
|
|
config := MustNewConfig(viper.GetViper())
|
|
if !ready(config) {
|
|
os.Exit(1)
|
|
}
|
|
},
|
|
}
|
|
}
|
|
|
|
func ready(config *Config) bool {
|
|
scheme := "https"
|
|
if !config.TLS.Enabled {
|
|
scheme = "http"
|
|
}
|
|
// Checking the TLS cert is not in the scope of the readiness check
|
|
httpClient := http.Client{Transport: &http.Transport{TLSClientConfig: &tls.Config{InsecureSkipVerify: true}}}
|
|
res, err := httpClient.Get(scheme + "://" + net.JoinHostPort("localhost", strconv.Itoa(int(config.Port))) + "/debug/ready")
|
|
if err != nil {
|
|
logging.WithError(err).Warn("ready check failed")
|
|
return false
|
|
}
|
|
defer res.Body.Close()
|
|
if res.StatusCode != 200 {
|
|
logging.WithFields("status", res.StatusCode).Warn("ready check failed")
|
|
return false
|
|
}
|
|
return true
|
|
}
|