mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
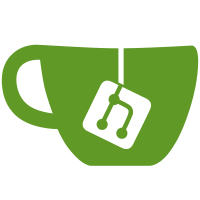
# Which Problems Are Solved As an administrator I want to be able to invite users to my application with the API V2, some user data I will already prefil, the user should add the authentication method themself (password, passkey, sso). # How the Problems Are Solved - A user can now be created with a email explicitly set to false. - If a user has no verified email and no authentication method, an `InviteCode` can be created through the User V2 API. - the code can be returned or sent through email - additionally `URLTemplate` and an `ApplicatioName` can provided for the email - The code can be resent and verified through the User V2 API - The V1 login allows users to verify and resend the code and set a password (analog user initialization) - The message text for the user invitation can be customized # Additional Changes - `verifyUserPasskeyCode` directly uses `crypto.VerifyCode` (instead of `verifyEncryptedCode`) - `verifyEncryptedCode` is removed (unnecessarily queried for the code generator) # Additional Context - closes #8310 - TODO: login V2 will have to implement invite flow: https://github.com/zitadel/typescript/issues/166
68 lines
2.2 KiB
Go
68 lines
2.2 KiB
Go
package domain
|
|
|
|
import (
|
|
"golang.org/x/text/language"
|
|
|
|
"github.com/zitadel/zitadel/internal/eventstore/v1/models"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
const (
|
|
InitCodeMessageType = "InitCode"
|
|
PasswordResetMessageType = "PasswordReset"
|
|
VerifyEmailMessageType = "VerifyEmail"
|
|
VerifyPhoneMessageType = "VerifyPhone"
|
|
VerifySMSOTPMessageType = "VerifySMSOTP"
|
|
VerifyEmailOTPMessageType = "VerifyEmailOTP"
|
|
DomainClaimedMessageType = "DomainClaimed"
|
|
PasswordlessRegistrationMessageType = "PasswordlessRegistration"
|
|
PasswordChangeMessageType = "PasswordChange"
|
|
InviteUserMessageType = "InviteUser"
|
|
MessageTitle = "Title"
|
|
MessagePreHeader = "PreHeader"
|
|
MessageSubject = "Subject"
|
|
MessageGreeting = "Greeting"
|
|
MessageText = "Text"
|
|
MessageButtonText = "ButtonText"
|
|
MessageFooterText = "Footer"
|
|
)
|
|
|
|
type CustomMessageText struct {
|
|
models.ObjectRoot
|
|
|
|
State PolicyState
|
|
Default bool
|
|
MessageTextType string
|
|
Language language.Tag
|
|
Title string
|
|
PreHeader string
|
|
Subject string
|
|
Greeting string
|
|
Text string
|
|
ButtonText string
|
|
FooterText string
|
|
}
|
|
|
|
func (m *CustomMessageText) IsValid(supportedLanguages []language.Tag) error {
|
|
if m.MessageTextType == "" {
|
|
return zerrors.ThrowInvalidArgument(nil, "INSTANCE-kd9fs", "Errors.CustomMessageText.Invalid")
|
|
}
|
|
if err := LanguageIsDefined(m.Language); err != nil {
|
|
return err
|
|
}
|
|
return LanguagesAreSupported(supportedLanguages, m.Language)
|
|
}
|
|
|
|
func IsMessageTextType(textType string) bool {
|
|
return textType == InitCodeMessageType ||
|
|
textType == PasswordResetMessageType ||
|
|
textType == VerifyEmailMessageType ||
|
|
textType == VerifyPhoneMessageType ||
|
|
textType == VerifySMSOTPMessageType ||
|
|
textType == VerifyEmailOTPMessageType ||
|
|
textType == DomainClaimedMessageType ||
|
|
textType == PasswordlessRegistrationMessageType ||
|
|
textType == PasswordChangeMessageType ||
|
|
textType == InviteUserMessageType
|
|
}
|