mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
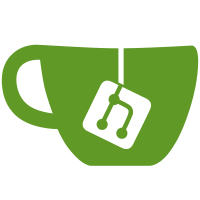
# Which Problems Are Solved As an administrator I want to be able to invite users to my application with the API V2, some user data I will already prefil, the user should add the authentication method themself (password, passkey, sso). # How the Problems Are Solved - A user can now be created with a email explicitly set to false. - If a user has no verified email and no authentication method, an `InviteCode` can be created through the User V2 API. - the code can be returned or sent through email - additionally `URLTemplate` and an `ApplicatioName` can provided for the email - The code can be resent and verified through the User V2 API - The V1 login allows users to verify and resend the code and set a password (analog user initialization) - The message text for the user invitation can be customized # Additional Changes - `verifyUserPasskeyCode` directly uses `crypto.VerifyCode` (instead of `verifyEncryptedCode`) - `verifyEncryptedCode` is removed (unnecessarily queried for the code generator) # Additional Context - closes #8310 - TODO: login V2 will have to implement invite flow: https://github.com/zitadel/typescript/issues/166
33 lines
854 B
Go
33 lines
854 B
Go
package domain
|
|
|
|
//go:generate enumer -type SecretGeneratorType -transform snake -trimprefix SecretGeneratorType
|
|
type SecretGeneratorType int32
|
|
|
|
const (
|
|
SecretGeneratorTypeUnspecified SecretGeneratorType = iota
|
|
SecretGeneratorTypeInitCode
|
|
SecretGeneratorTypeVerifyEmailCode
|
|
SecretGeneratorTypeVerifyPhoneCode
|
|
SecretGeneratorTypeVerifyDomain
|
|
SecretGeneratorTypePasswordResetCode
|
|
SecretGeneratorTypePasswordlessInitCode
|
|
SecretGeneratorTypeAppSecret
|
|
SecretGeneratorTypeOTPSMS
|
|
SecretGeneratorTypeOTPEmail
|
|
SecretGeneratorTypeInviteCode
|
|
|
|
secretGeneratorTypeCount
|
|
)
|
|
|
|
func (t SecretGeneratorType) Valid() bool {
|
|
return t > SecretGeneratorTypeUnspecified && t < secretGeneratorTypeCount
|
|
}
|
|
|
|
type SecretGeneratorState int32
|
|
|
|
const (
|
|
SecretGeneratorStateUnspecified SecretGeneratorState = iota
|
|
SecretGeneratorStateActive
|
|
SecretGeneratorStateRemoved
|
|
)
|