mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
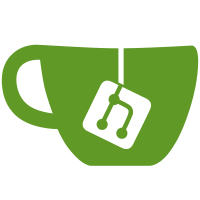
* add token exchange feature flag * allow setting reason and actor to access tokens * impersonation * set token types and scopes in response * upgrade oidc to working draft state * fix tests * audience and scope validation * id toke and jwt as input * return id tokens * add grant type token exchange to app config * add integration tests * check and deny actors in api calls * fix instance setting tests by triggering projection on write and cleanup * insert sleep statements again * solve linting issues * add translations * pin oidc v3.15.0 * resolve comments, add event translation * fix refreshtoken test * use ValidateAuthReqScopes from oidc * apparently the linter can't make up its mind * persist actor thru refresh tokens and check in tests * remove unneeded triggers
117 lines
3.8 KiB
Go
117 lines
3.8 KiB
Go
// Code generated by "enumer -type TokenReason -transform snake -trimprefix TokenReason -json"; DO NOT EDIT.
|
|
|
|
package domain
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
const _TokenReasonName = "unspecifiedauth_requestrefreshjwt_profileclient_credentialsexchangeimpersonation"
|
|
|
|
var _TokenReasonIndex = [...]uint8{0, 11, 23, 30, 41, 59, 67, 80}
|
|
|
|
const _TokenReasonLowerName = "unspecifiedauth_requestrefreshjwt_profileclient_credentialsexchangeimpersonation"
|
|
|
|
func (i TokenReason) String() string {
|
|
if i < 0 || i >= TokenReason(len(_TokenReasonIndex)-1) {
|
|
return fmt.Sprintf("TokenReason(%d)", i)
|
|
}
|
|
return _TokenReasonName[_TokenReasonIndex[i]:_TokenReasonIndex[i+1]]
|
|
}
|
|
|
|
// An "invalid array index" compiler error signifies that the constant values have changed.
|
|
// Re-run the stringer command to generate them again.
|
|
func _TokenReasonNoOp() {
|
|
var x [1]struct{}
|
|
_ = x[TokenReasonUnspecified-(0)]
|
|
_ = x[TokenReasonAuthRequest-(1)]
|
|
_ = x[TokenReasonRefresh-(2)]
|
|
_ = x[TokenReasonJWTProfile-(3)]
|
|
_ = x[TokenReasonClientCredentials-(4)]
|
|
_ = x[TokenReasonExchange-(5)]
|
|
_ = x[TokenReasonImpersonation-(6)]
|
|
}
|
|
|
|
var _TokenReasonValues = []TokenReason{TokenReasonUnspecified, TokenReasonAuthRequest, TokenReasonRefresh, TokenReasonJWTProfile, TokenReasonClientCredentials, TokenReasonExchange, TokenReasonImpersonation}
|
|
|
|
var _TokenReasonNameToValueMap = map[string]TokenReason{
|
|
_TokenReasonName[0:11]: TokenReasonUnspecified,
|
|
_TokenReasonLowerName[0:11]: TokenReasonUnspecified,
|
|
_TokenReasonName[11:23]: TokenReasonAuthRequest,
|
|
_TokenReasonLowerName[11:23]: TokenReasonAuthRequest,
|
|
_TokenReasonName[23:30]: TokenReasonRefresh,
|
|
_TokenReasonLowerName[23:30]: TokenReasonRefresh,
|
|
_TokenReasonName[30:41]: TokenReasonJWTProfile,
|
|
_TokenReasonLowerName[30:41]: TokenReasonJWTProfile,
|
|
_TokenReasonName[41:59]: TokenReasonClientCredentials,
|
|
_TokenReasonLowerName[41:59]: TokenReasonClientCredentials,
|
|
_TokenReasonName[59:67]: TokenReasonExchange,
|
|
_TokenReasonLowerName[59:67]: TokenReasonExchange,
|
|
_TokenReasonName[67:80]: TokenReasonImpersonation,
|
|
_TokenReasonLowerName[67:80]: TokenReasonImpersonation,
|
|
}
|
|
|
|
var _TokenReasonNames = []string{
|
|
_TokenReasonName[0:11],
|
|
_TokenReasonName[11:23],
|
|
_TokenReasonName[23:30],
|
|
_TokenReasonName[30:41],
|
|
_TokenReasonName[41:59],
|
|
_TokenReasonName[59:67],
|
|
_TokenReasonName[67:80],
|
|
}
|
|
|
|
// TokenReasonString retrieves an enum value from the enum constants string name.
|
|
// Throws an error if the param is not part of the enum.
|
|
func TokenReasonString(s string) (TokenReason, error) {
|
|
if val, ok := _TokenReasonNameToValueMap[s]; ok {
|
|
return val, nil
|
|
}
|
|
|
|
if val, ok := _TokenReasonNameToValueMap[strings.ToLower(s)]; ok {
|
|
return val, nil
|
|
}
|
|
return 0, fmt.Errorf("%s does not belong to TokenReason values", s)
|
|
}
|
|
|
|
// TokenReasonValues returns all values of the enum
|
|
func TokenReasonValues() []TokenReason {
|
|
return _TokenReasonValues
|
|
}
|
|
|
|
// TokenReasonStrings returns a slice of all String values of the enum
|
|
func TokenReasonStrings() []string {
|
|
strs := make([]string, len(_TokenReasonNames))
|
|
copy(strs, _TokenReasonNames)
|
|
return strs
|
|
}
|
|
|
|
// IsATokenReason returns "true" if the value is listed in the enum definition. "false" otherwise
|
|
func (i TokenReason) IsATokenReason() bool {
|
|
for _, v := range _TokenReasonValues {
|
|
if i == v {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
// MarshalJSON implements the json.Marshaler interface for TokenReason
|
|
func (i TokenReason) MarshalJSON() ([]byte, error) {
|
|
return json.Marshal(i.String())
|
|
}
|
|
|
|
// UnmarshalJSON implements the json.Unmarshaler interface for TokenReason
|
|
func (i *TokenReason) UnmarshalJSON(data []byte) error {
|
|
var s string
|
|
if err := json.Unmarshal(data, &s); err != nil {
|
|
return fmt.Errorf("TokenReason should be a string, got %s", data)
|
|
}
|
|
|
|
var err error
|
|
*i, err = TokenReasonString(s)
|
|
return err
|
|
}
|