mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
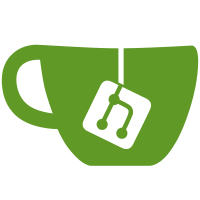
# Which Problems Are Solved As already mentioned and (partially) fixed in #7992 we discovered, issues with v2 tokens that where obtained through an IDP, with passwordless authentication or with password authentication (wihtout any 2FA set up) using the v1 login for zitadel API calls - (Previous) authentication through an IdP is now correctly treated as auth method in case of a reauth even when the user is not redirected to the IdP - There were some cases where passwordless authentication was successfully checked but not correctly set as auth method, which denied access to ZITADEL API - Users with password and passwordless, but no 2FA set up which authenticate just wich password can access the ZITADEL API again Additionally while testing we found out that because of #7969 the login UI could completely break / block with the following error: `sql: Scan error on column index 3, name "state": converting NULL to int32 is unsupported (Internal)` # How the Problems Are Solved - IdP checks are treated the same way as other factors and it's ensured that a succeeded check within the configured timeframe will always provide the idp auth method - `MFATypesAllowed` checks for possible passwordless authentication - As with the v1 login, the token check now only requires MFA if the policy is set or the user has 2FA set up - UserAuthMethodsRequirements now always uses the correctly policy to check for MFA enforcement - `State` column is handled as nullable and additional events set the state to active (as before #7969) # Additional Changes - Console now also checks for 403 (mfa required) errors (e.g. after setting up the first 2FA in console) and redirects the user to the login UI (with the current id_token as id_token_hint) - Possible duplicates in auth methods / AMRs are removed now as well. # Additional Context - Bugs were introduced in #7822 and # and 7969 and only part of a pre-release. - partially already fixed with #7992 - Reported internally.
123 lines
3.1 KiB
Go
123 lines
3.1 KiB
Go
package domain
|
|
|
|
type UserState int32
|
|
|
|
const (
|
|
UserStateUnspecified UserState = iota
|
|
UserStateActive
|
|
UserStateInactive
|
|
UserStateDeleted
|
|
UserStateLocked
|
|
UserStateSuspend
|
|
UserStateInitial
|
|
|
|
userStateCount
|
|
)
|
|
|
|
func (s UserState) Exists() bool {
|
|
return s != UserStateUnspecified && s != UserStateDeleted
|
|
}
|
|
|
|
func (s UserState) IsEnabled() bool {
|
|
return s == UserStateActive || s == UserStateInitial
|
|
}
|
|
|
|
type UserType int32
|
|
|
|
const (
|
|
UserTypeUnspecified UserType = iota
|
|
UserTypeHuman
|
|
UserTypeMachine
|
|
userTypeCount
|
|
)
|
|
|
|
type UserAuthMethodType int32
|
|
|
|
const (
|
|
UserAuthMethodTypeUnspecified UserAuthMethodType = iota
|
|
UserAuthMethodTypeTOTP
|
|
UserAuthMethodTypeU2F
|
|
UserAuthMethodTypePasswordless
|
|
UserAuthMethodTypePassword
|
|
UserAuthMethodTypeIDP
|
|
UserAuthMethodTypeOTPSMS
|
|
UserAuthMethodTypeOTPEmail
|
|
UserAuthMethodTypeOTP // generic OTP when parsing AMR from OIDC
|
|
UserAuthMethodTypePrivateKey
|
|
userAuthMethodTypeCount
|
|
)
|
|
|
|
// HasMFA checks whether the user authenticated with multiple auth factors.
|
|
// This can either be true if the list contains a [UserAuthMethodType] which by itself is MFA (e.g. [UserAuthMethodTypePasswordless])
|
|
// or if multiple factors were used (e.g. [UserAuthMethodTypePassword] and [UserAuthMethodTypeU2F])
|
|
func HasMFA(methods []UserAuthMethodType) bool {
|
|
var factors int
|
|
for _, method := range methods {
|
|
switch method {
|
|
case UserAuthMethodTypePasswordless:
|
|
return true
|
|
case UserAuthMethodTypePassword,
|
|
UserAuthMethodTypeU2F,
|
|
UserAuthMethodTypeTOTP,
|
|
UserAuthMethodTypeOTPSMS,
|
|
UserAuthMethodTypeOTPEmail,
|
|
UserAuthMethodTypeIDP,
|
|
UserAuthMethodTypeOTP,
|
|
UserAuthMethodTypePrivateKey:
|
|
factors++
|
|
case UserAuthMethodTypeUnspecified,
|
|
userAuthMethodTypeCount:
|
|
// ignore
|
|
}
|
|
}
|
|
return factors > 1
|
|
}
|
|
|
|
// Has2FA checks whether the auth factors provided are a second factor and will return true if at least one is.
|
|
func Has2FA(methods []UserAuthMethodType) bool {
|
|
var factors int
|
|
for _, method := range methods {
|
|
switch method {
|
|
case
|
|
UserAuthMethodTypeU2F,
|
|
UserAuthMethodTypeTOTP,
|
|
UserAuthMethodTypeOTPSMS,
|
|
UserAuthMethodTypeOTPEmail,
|
|
UserAuthMethodTypeOTP:
|
|
factors++
|
|
case UserAuthMethodTypeUnspecified,
|
|
UserAuthMethodTypePassword,
|
|
UserAuthMethodTypePasswordless,
|
|
UserAuthMethodTypeIDP,
|
|
UserAuthMethodTypePrivateKey,
|
|
userAuthMethodTypeCount:
|
|
// ignore
|
|
}
|
|
}
|
|
return factors > 0
|
|
}
|
|
|
|
// RequiresMFA checks whether the user requires to authenticate with multiple auth factors based on the LoginPolicy and the authentication type.
|
|
// Internal authentication will require MFA if either option is activated.
|
|
// External authentication will only require MFA if it's forced generally and not local only.
|
|
func RequiresMFA(forceMFA, forceMFALocalOnly, isInternalLogin bool) bool {
|
|
if isInternalLogin {
|
|
return forceMFA || forceMFALocalOnly
|
|
}
|
|
return forceMFA && !forceMFALocalOnly
|
|
}
|
|
|
|
type PersonalAccessTokenState int32
|
|
|
|
const (
|
|
PersonalAccessTokenStateUnspecified PersonalAccessTokenState = iota
|
|
PersonalAccessTokenStateActive
|
|
PersonalAccessTokenStateRemoved
|
|
|
|
personalAccessTokenStateCount
|
|
)
|
|
|
|
func (f PersonalAccessTokenState) Valid() bool {
|
|
return f >= 0 && f < personalAccessTokenStateCount
|
|
}
|