mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 08:49:13 +00:00
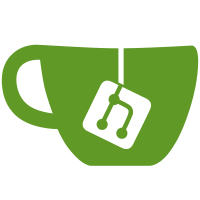
* feat: jwt idp * feat: command side * feat: add tests * fill idp views with jwt idps and return apis * add jwtEndpoint to jwt idp * begin jwt request handling * merge * handle jwt idp * cleanup * fixes * autoregister * get token from specific header name * error handling * fix texts * handle renderExternalNotFoundOption Co-authored-by: fabi <fabienne.gerschwiler@gmail.com>
127 lines
2.6 KiB
Go
127 lines
2.6 KiB
Go
package domain
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/caos/zitadel/internal/crypto"
|
|
es_models "github.com/caos/zitadel/internal/eventstore/v1/models"
|
|
)
|
|
|
|
type IDPConfig struct {
|
|
es_models.ObjectRoot
|
|
IDPConfigID string
|
|
Type IDPConfigType
|
|
Name string
|
|
StylingType IDPConfigStylingType
|
|
State IDPConfigState
|
|
OIDCConfig *OIDCIDPConfig
|
|
JWTConfig *JWTIDPConfig
|
|
AutoRegister bool
|
|
}
|
|
|
|
type IDPConfigView struct {
|
|
AggregateID string
|
|
IDPConfigID string
|
|
Name string
|
|
StylingType IDPConfigStylingType
|
|
State IDPConfigState
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
Sequence uint64
|
|
IDPProviderType IdentityProviderType
|
|
AutoRegister bool
|
|
|
|
IsOIDC bool
|
|
OIDCClientID string
|
|
OIDCClientSecret *crypto.CryptoValue
|
|
OIDCIssuer string
|
|
OIDCScopes []string
|
|
OIDCIDPDisplayNameMapping OIDCMappingField
|
|
OIDCUsernameMapping OIDCMappingField
|
|
OAuthAuthorizationEndpoint string
|
|
OAuthTokenEndpoint string
|
|
|
|
JWTEndpoint string
|
|
JWTIssuer string
|
|
JWTKeysEndpoint string
|
|
}
|
|
|
|
type OIDCIDPConfig struct {
|
|
es_models.ObjectRoot
|
|
IDPConfigID string
|
|
ClientID string
|
|
ClientSecret *crypto.CryptoValue
|
|
ClientSecretString string
|
|
Issuer string
|
|
AuthorizationEndpoint string
|
|
TokenEndpoint string
|
|
Scopes []string
|
|
IDPDisplayNameMapping OIDCMappingField
|
|
UsernameMapping OIDCMappingField
|
|
}
|
|
|
|
type JWTIDPConfig struct {
|
|
es_models.ObjectRoot
|
|
IDPConfigID string
|
|
JWTEndpoint string
|
|
Issuer string
|
|
KeysEndpoint string
|
|
HeaderName string
|
|
}
|
|
|
|
type IDPConfigType int32
|
|
|
|
const (
|
|
IDPConfigTypeOIDC IDPConfigType = iota
|
|
IDPConfigTypeSAML
|
|
IDPConfigTypeJWT
|
|
|
|
//count is for validation
|
|
idpConfigTypeCount
|
|
)
|
|
|
|
func (f IDPConfigType) Valid() bool {
|
|
return f >= 0 && f < idpConfigTypeCount
|
|
}
|
|
|
|
type IDPConfigState int32
|
|
|
|
const (
|
|
IDPConfigStateUnspecified IDPConfigState = iota
|
|
IDPConfigStateActive
|
|
IDPConfigStateInactive
|
|
IDPConfigStateRemoved
|
|
|
|
idpConfigStateCount
|
|
)
|
|
|
|
func (s IDPConfigState) Valid() bool {
|
|
return s >= 0 && s < idpConfigStateCount
|
|
}
|
|
|
|
func (s IDPConfigState) Exists() bool {
|
|
return s != IDPConfigStateUnspecified || s == IDPConfigStateRemoved
|
|
}
|
|
|
|
type IDPConfigStylingType int32
|
|
|
|
const (
|
|
IDPConfigStylingTypeUnspecified IDPConfigStylingType = iota
|
|
IDPConfigStylingTypeGoogle
|
|
|
|
idpConfigStylingTypeCount
|
|
)
|
|
|
|
func (f IDPConfigStylingType) Valid() bool {
|
|
return f >= 0 && f < idpConfigStylingTypeCount
|
|
}
|
|
|
|
func (st IDPConfigStylingType) GetCSSClass() string {
|
|
switch st {
|
|
case IDPConfigStylingTypeGoogle:
|
|
return "google"
|
|
default:
|
|
return ""
|
|
}
|
|
}
|