mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 18:44:40 +00:00
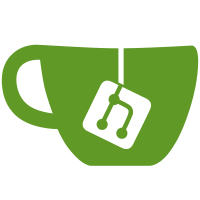
* feat: default custom message text * feat: org custom message text * feat: org custom message text * feat: custom messages query side * feat: default messages * feat: message text user fields * feat: check for inactive user * feat: fix send password reset * feat: fix custom org text * feat: add variables to docs * feat: custom text tests * feat: fix notifications * feat: add custom text feature * feat: add custom text feature * feat: feature in custom message texts * feat: add custom text feature in frontend * feat: merge main * feat: feature tests * feat: change phone message in setup * fix: remove unused code, add event translation * fix: merge main and fix problems * fix: english translation file * fix: migration versions * fix: setup * feat: fix pr requests * feat: fix phone code message * feat: migration * feat: setup * fix: remove unused tests Co-authored-by: Livio Amstutz <livio.a@gmail.com>
91 lines
2.4 KiB
Go
91 lines
2.4 KiB
Go
package model
|
|
|
|
import (
|
|
"github.com/caos/zitadel/internal/domain"
|
|
caos_errors "github.com/caos/zitadel/internal/errors"
|
|
|
|
"time"
|
|
)
|
|
|
|
type ProjectGrantView struct {
|
|
ProjectID string
|
|
Name string
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
State ProjectState
|
|
ResourceOwner string
|
|
ResourceOwnerName string
|
|
OrgID string
|
|
OrgName string
|
|
OrgDomain string
|
|
Sequence uint64
|
|
GrantID string
|
|
GrantedRoleKeys []string
|
|
}
|
|
|
|
type ProjectGrantViewSearchRequest struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
SortingColumn ProjectGrantViewSearchKey
|
|
Asc bool
|
|
Queries []*ProjectGrantViewSearchQuery
|
|
}
|
|
|
|
type ProjectGrantViewSearchKey int32
|
|
|
|
const (
|
|
GrantedProjectSearchKeyUnspecified ProjectGrantViewSearchKey = iota
|
|
GrantedProjectSearchKeyName
|
|
GrantedProjectSearchKeyProjectID
|
|
GrantedProjectSearchKeyGrantID
|
|
GrantedProjectSearchKeyOrgID
|
|
GrantedProjectSearchKeyResourceOwner
|
|
GrantedProjectSearchKeyRoleKeys
|
|
)
|
|
|
|
type ProjectGrantViewSearchQuery struct {
|
|
Key ProjectGrantViewSearchKey
|
|
Method domain.SearchMethod
|
|
Value interface{}
|
|
}
|
|
|
|
type ProjectGrantViewSearchResponse struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
TotalResult uint64
|
|
Result []*ProjectGrantView
|
|
Sequence uint64
|
|
Timestamp time.Time
|
|
}
|
|
|
|
func (r *ProjectGrantViewSearchRequest) GetSearchQuery(key ProjectGrantViewSearchKey) (int, *ProjectGrantViewSearchQuery) {
|
|
for i, q := range r.Queries {
|
|
if q.Key == key {
|
|
return i, q
|
|
}
|
|
}
|
|
return -1, nil
|
|
}
|
|
|
|
func (r *ProjectGrantViewSearchRequest) AppendMyOrgQuery(orgID string) {
|
|
r.Queries = append(r.Queries, &ProjectGrantViewSearchQuery{Key: GrantedProjectSearchKeyOrgID, Method: domain.SearchMethodEquals, Value: orgID})
|
|
}
|
|
|
|
func (r *ProjectGrantViewSearchRequest) AppendNotMyOrgQuery(orgID string) {
|
|
r.Queries = append(r.Queries, &ProjectGrantViewSearchQuery{Key: GrantedProjectSearchKeyOrgID, Method: domain.SearchMethodNotEquals, Value: orgID})
|
|
}
|
|
|
|
func (r *ProjectGrantViewSearchRequest) AppendMyResourceOwnerQuery(orgID string) {
|
|
r.Queries = append(r.Queries, &ProjectGrantViewSearchQuery{Key: GrantedProjectSearchKeyResourceOwner, Method: domain.SearchMethodEquals, Value: orgID})
|
|
}
|
|
|
|
func (r *ProjectGrantViewSearchRequest) EnsureLimit(limit uint64) error {
|
|
if r.Limit > limit {
|
|
return caos_errors.ThrowInvalidArgument(nil, "SEARCH-0fj3s", "Errors.Limit.ExceedsDefault")
|
|
}
|
|
if r.Limit == 0 {
|
|
r.Limit = limit
|
|
}
|
|
return nil
|
|
}
|