mirror of
https://github.com/zitadel/zitadel
synced 2024-11-21 16:30:53 +00:00
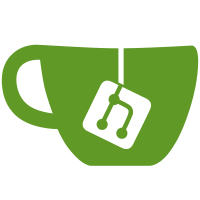
# Which Problems Are Solved The mirror command read the configurations in the wrong order # How the Problems Are Solved The Pre execution run of `mirror` reads the default config first and then applies the custom configs
76 lines
1.8 KiB
Go
76 lines
1.8 KiB
Go
package cmd
|
|
|
|
import (
|
|
"bytes"
|
|
_ "embed"
|
|
"errors"
|
|
"io"
|
|
"strings"
|
|
|
|
"github.com/spf13/cobra"
|
|
"github.com/spf13/viper"
|
|
"github.com/zitadel/logging"
|
|
|
|
"github.com/zitadel/zitadel/cmd/admin"
|
|
"github.com/zitadel/zitadel/cmd/build"
|
|
"github.com/zitadel/zitadel/cmd/initialise"
|
|
"github.com/zitadel/zitadel/cmd/key"
|
|
"github.com/zitadel/zitadel/cmd/mirror"
|
|
"github.com/zitadel/zitadel/cmd/ready"
|
|
"github.com/zitadel/zitadel/cmd/setup"
|
|
"github.com/zitadel/zitadel/cmd/start"
|
|
)
|
|
|
|
var (
|
|
configFiles []string
|
|
|
|
//go:embed defaults.yaml
|
|
defaultConfig []byte
|
|
)
|
|
|
|
func New(out io.Writer, in io.Reader, args []string, server chan<- *start.Server) *cobra.Command {
|
|
cmd := &cobra.Command{
|
|
Use: "zitadel",
|
|
Short: "The ZITADEL CLI lets you interact with ZITADEL",
|
|
Long: `The ZITADEL CLI lets you interact with ZITADEL`,
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
return errors.New("no additional command provided")
|
|
},
|
|
Version: build.Version(),
|
|
}
|
|
|
|
viper.AutomaticEnv()
|
|
viper.SetEnvPrefix("ZITADEL")
|
|
viper.SetEnvKeyReplacer(strings.NewReplacer(".", "_"))
|
|
viper.SetConfigType("yaml")
|
|
err := viper.ReadConfig(bytes.NewBuffer(defaultConfig))
|
|
logging.OnError(err).Fatal("unable to read default config")
|
|
|
|
cobra.OnInitialize(initConfig)
|
|
cmd.PersistentFlags().StringArrayVar(&configFiles, "config", nil, "path to config file to overwrite system defaults")
|
|
|
|
cmd.AddCommand(
|
|
admin.New(), //is now deprecated, remove later on
|
|
initialise.New(),
|
|
setup.New(),
|
|
start.New(server),
|
|
start.NewStartFromInit(server),
|
|
start.NewStartFromSetup(server),
|
|
mirror.New(&configFiles),
|
|
key.New(),
|
|
ready.New(),
|
|
)
|
|
|
|
cmd.InitDefaultVersionFlag()
|
|
|
|
return cmd
|
|
}
|
|
|
|
func initConfig() {
|
|
for _, file := range configFiles {
|
|
viper.SetConfigFile(file)
|
|
err := viper.MergeInConfig()
|
|
logging.WithFields("file", file).OnError(err).Warn("unable to read config file")
|
|
}
|
|
}
|