mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
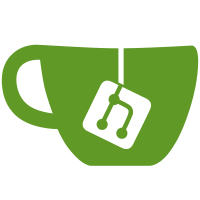
# Which Problems Are Solved We identified the need of caching. Currently we have a number of places where we use different ways of caching, like go maps or LRU. We might also want shared chaches in the future, like Redis-based or in special SQL tables. # How the Problems Are Solved Define a generic Cache interface which allows different implementations. - A noop implementation is provided and enabled as. - An implementation using go maps is provided - disabled in defaults.yaml - enabled in integration tests - Authz middleware instance objects are cached using the interface. # Additional Changes - Enabled integration test command raceflag - Fix a race condition in the limits integration test client - Fix a number of flaky integration tests. (Because zitadel is super fast now!) 🎸 🚀 # Additional Context Related to https://github.com/zitadel/zitadel/issues/8648
77 lines
1.9 KiB
Go
77 lines
1.9 KiB
Go
package cache
|
|
|
|
import (
|
|
"context"
|
|
"math/rand"
|
|
"time"
|
|
|
|
"github.com/jonboulle/clockwork"
|
|
"github.com/zitadel/logging"
|
|
)
|
|
|
|
// Pruner is an optional [Cache] interface.
|
|
type Pruner interface {
|
|
// Prune deletes all invalidated or expired objects.
|
|
Prune(ctx context.Context) error
|
|
}
|
|
|
|
type PrunerCache[I, K comparable, V Entry[I, K]] interface {
|
|
Cache[I, K, V]
|
|
Pruner
|
|
}
|
|
|
|
type AutoPruneConfig struct {
|
|
// Interval at which the cache is automatically pruned.
|
|
// 0 or lower disables automatic pruning.
|
|
Interval time.Duration
|
|
|
|
// Timeout for an automatic prune.
|
|
// It is recommended to keep the value shorter than AutoPruneInterval
|
|
// 0 or lower disables automatic pruning.
|
|
Timeout time.Duration
|
|
}
|
|
|
|
func (c AutoPruneConfig) StartAutoPrune(background context.Context, pruner Pruner, name string) (close func()) {
|
|
return c.startAutoPrune(background, pruner, name, clockwork.NewRealClock())
|
|
}
|
|
|
|
func (c *AutoPruneConfig) startAutoPrune(background context.Context, pruner Pruner, name string, clock clockwork.Clock) (close func()) {
|
|
if c.Interval <= 0 {
|
|
return func() {}
|
|
}
|
|
background, cancel := context.WithCancel(background)
|
|
// randomize the first interval
|
|
timer := clock.NewTimer(time.Duration(rand.Int63n(int64(c.Interval))))
|
|
go c.pruneTimer(background, pruner, name, timer)
|
|
return cancel
|
|
}
|
|
|
|
func (c *AutoPruneConfig) pruneTimer(background context.Context, pruner Pruner, name string, timer clockwork.Timer) {
|
|
defer func() {
|
|
if !timer.Stop() {
|
|
<-timer.Chan()
|
|
}
|
|
}()
|
|
|
|
for {
|
|
select {
|
|
case <-background.Done():
|
|
return
|
|
case <-timer.Chan():
|
|
timer.Reset(c.Interval)
|
|
err := c.doPrune(background, pruner)
|
|
logging.OnError(err).WithField("name", name).Error("cache auto prune")
|
|
}
|
|
}
|
|
}
|
|
|
|
func (c *AutoPruneConfig) doPrune(background context.Context, pruner Pruner) error {
|
|
ctx, cancel := context.WithCancel(background)
|
|
defer cancel()
|
|
if c.Timeout > 0 {
|
|
ctx, cancel = context.WithTimeout(background, c.Timeout)
|
|
defer cancel()
|
|
}
|
|
return pruner.Prune(ctx)
|
|
}
|