mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
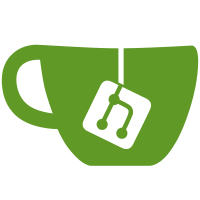
* feat(crypto): use passwap for machine and app secrets * fix command package tests * add hash generator command test * naming convention, fix query tests * rename PasswordHasher and cleanup start commands * add reducer tests * fix intergration tests, cleanup old config * add app secret unit tests * solve setup panics * fix push of updated events * add missing event translations * update documentation * solve linter errors * remove nolint:SA1019 as it doesn't seem to help anyway * add nolint to deprecated filter usage * update users migration version * remove unused ClientSecret from APIConfigChangedEvent --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
65 lines
1.5 KiB
Go
65 lines
1.5 KiB
Go
package domain
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
es_models "github.com/zitadel/zitadel/internal/eventstore/v1/models"
|
|
"github.com/zitadel/zitadel/internal/telemetry/tracing"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
type Password struct {
|
|
es_models.ObjectRoot
|
|
|
|
SecretString string
|
|
EncodedSecret string
|
|
ChangeRequired bool
|
|
}
|
|
|
|
func NewPassword(password string) *Password {
|
|
return &Password{
|
|
SecretString: password,
|
|
}
|
|
}
|
|
|
|
type PasswordCode struct {
|
|
es_models.ObjectRoot
|
|
|
|
Code *crypto.CryptoValue
|
|
Expiry time.Duration
|
|
NotificationType NotificationType
|
|
}
|
|
|
|
func (p *Password) HashPasswordIfExisting(ctx context.Context, policy *PasswordComplexityPolicy, hasher *crypto.Hasher) error {
|
|
if p.SecretString == "" {
|
|
return nil
|
|
}
|
|
if policy == nil {
|
|
return zerrors.ThrowPreconditionFailed(nil, "DOMAIN-s8ifS", "Errors.User.PasswordComplexityPolicy.NotFound")
|
|
}
|
|
if err := policy.Check(p.SecretString); err != nil {
|
|
return err
|
|
}
|
|
_, spanHash := tracing.NewNamedSpan(ctx, "passwap.Hash")
|
|
encoded, err := hasher.Hash(p.SecretString)
|
|
spanHash.EndWithError(err)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
p.EncodedSecret = encoded
|
|
return nil
|
|
}
|
|
|
|
func NewPasswordCode(passwordGenerator crypto.Generator) (*PasswordCode, error) {
|
|
passwordCodeCrypto, _, err := crypto.NewCode(passwordGenerator)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &PasswordCode{
|
|
Code: passwordCodeCrypto,
|
|
Expiry: passwordGenerator.Expiry(),
|
|
}, nil
|
|
}
|