mirror of
https://github.com/zitadel/zitadel
synced 2024-11-22 00:39:36 +00:00
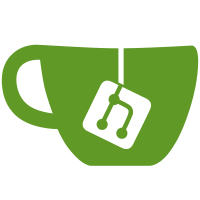
# Which Problems Are Solved Org v2 service does not have a ListOrganizations endpoint. # How the Problems Are Solved Implement ListOrganizations endpoint. # Additional Changes - moved descriptions in the protos to comments - corrected the RemoveNoPermissions for the ListUsers, to get the correct TotalResults # Additional Context For new typescript login
40 lines
1.1 KiB
Go
40 lines
1.1 KiB
Go
package domain
|
|
|
|
import "context"
|
|
|
|
type Permissions struct {
|
|
Permissions []string
|
|
}
|
|
|
|
func (p *Permissions) AppendPermissions(ctxID string, permissions ...string) {
|
|
for _, permission := range permissions {
|
|
p.appendPermission(ctxID, permission)
|
|
}
|
|
}
|
|
|
|
func (p *Permissions) appendPermission(ctxID, permission string) {
|
|
if ctxID != "" {
|
|
permission = permission + ":" + ctxID
|
|
}
|
|
for _, existingPermission := range p.Permissions {
|
|
if existingPermission == permission {
|
|
return
|
|
}
|
|
}
|
|
p.Permissions = append(p.Permissions, permission)
|
|
}
|
|
|
|
type PermissionCheck func(ctx context.Context, permission, orgID, resourceID string) (err error)
|
|
|
|
const (
|
|
PermissionUserWrite = "user.write"
|
|
PermissionUserRead = "user.read"
|
|
PermissionUserDelete = "user.delete"
|
|
PermissionUserCredentialWrite = "user.credential.write"
|
|
PermissionSessionWrite = "session.write"
|
|
PermissionSessionDelete = "session.delete"
|
|
PermissionOrgRead = "org.read"
|
|
PermissionIDPRead = "iam.idp.read"
|
|
PermissionOrgIDPRead = "org.idp.read"
|
|
)
|