mirror of
https://github.com/nocobase/nocobase
synced 2024-11-15 18:37:04 +00:00
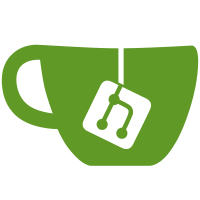
* refactor: plugin manager page
* fix: bug
* feat: addByNpm api
* fix: improve the addByNpm
* feat: improve applicationPlugins:list api
* fix: re-download npm package when restart app
* fix: plugin delete api
* feat: plugin detail api
* feat: zipUrl add api
* fix: upload api bug
* fix: plugin detail info
* feat: upgrade api
* fix: upload api
* feat: handle plugin load error
* feat: support authToken
* feat: muti lang
* fix: build error
* fix: self review
* Update plugin-manager.ts
* fix: bug
* fix: bug
* fix: bug
* fix: bug
* fix: bug
* fix: bugs
* fix: detail click and remove isOfficial
* fix: upgrade no refresh
* fix: file size and type check
* fix: bug
* fix: upgrade error
* fix: bug
* fix: bug
* fix: plugin card layout
* fix: handling exceptional cases
* fix: tgz file support
* fix: macos compress file
* fix: bug
* fix: bug
* fix: bug
* fix: bug
* fix: add upgrade npm type
* fix: bugs
* fix: bug
* fix: change plugins static expose url
* fix: api prefix
* fix: bug
* fix: add nginx `/static/plugin/` path
* fix: bugs and pr docker build no dts
* fix: bug
* fix: build tools bug
* fix: improve code
* fix: build bug
* feat: improve plugin info
* fix: ui bug
* fix: plugin document bug
* feat: improve code
* feat: improve code
* feat: process dev deps check
* feat: improve code
* feat: process.env.IS_DEV_CMD
* fix: do not delete the plugin package
* feat: plugin symlink
* fix: tsx watch --ignore=./storage/plugins/**
* fix: test error
* fix: improve code
* fix: improve code
* fix: emitStartedEvent
* fix: improve code
* fix: type error
* fix: test error
* test: console.log
* fix: createStoragePluginSymLink
* fix: clientStaticMiddleware rename to clientStaticUtils
* feat: build tools support plugins folder
* fix: 350px
* fix: error
* feat: client dev support plugin folder
* fix: clear cli options
* fix: typeError: Converting circular structure to JSON
* fix: plugin name
* chore: restart application after command
* feat: upgrade error & docs
* Update v14-changelog.md
* Update v14-changelog.md
* Update v14-changelog.md
* fix: gateway test
* refactor(plugin-workflow): add ready state for gracefully tearing down
* Revert "chore: restart application after command"
This reverts commit 5015274f8e
.
* chore: stop application whe restart
* T 1218 change plugin folder (#2629)
* feat: change folder name
* feat: change `pm create` command
* feat: revert plugin name change
* fix: delete samples
* feat: change plugins folder
* fix: pm create
* feat: update docs
* fix: link package error
* fix: docs
* fix: create command
* fix: pm add error
* fix: create add build
* fix: pm creatre + add
* feat: add tar command
* fix: docs
* fix: bug
* fix: docs
---------
Co-authored-by: chenos <chenlinxh@gmail.com>
* feat: docs
* Update your-fisrt-plugin.md
* Update your-fisrt-plugin.md
* chore: application reload
* chore: test
* fix: pm add error
* chore: preset install skip exists plugin
* fix: createIfNotExists
---------
Co-authored-by: chenos <chenlinxh@gmail.com>
Co-authored-by: chareice <chareice@live.com>
Co-authored-by: Zhou <zhou.working@gmail.com>
Co-authored-by: mytharcher <mytharcher@gmail.com>
137 lines
3.0 KiB
Markdown
137 lines
3.0 KiB
Markdown
# 编写第一个插件
|
||
|
||
在开始前,需要先安装好 NocoBase:
|
||
|
||
- [create-nocobase-app 安装](/welcome/getting-started/installation/create-nocobase-app)
|
||
- [Git 源码安装](/welcome/getting-started/installation/git-clone)
|
||
|
||
安装好 NocoBase 之后,我们就可以开始插件开发之旅了。
|
||
|
||
## 创建插件
|
||
|
||
通过 CLI 快速地创建一个空插件,命令如下:
|
||
|
||
```bash
|
||
yarn pm create @my-project/plugin-hello
|
||
```
|
||
|
||
插件所在目录 `packages/plugins/@my-project/plugin-hello`,插件目录结构为:
|
||
|
||
```bash
|
||
|- /packages/plugins/@my-project/plugin-hello
|
||
|- /src
|
||
|- /client # 插件客户端代码
|
||
|- /server # 插件服务端代码
|
||
|- client.d.ts
|
||
|- client.js
|
||
|- package.json # 插件包信息
|
||
|- server.d.ts
|
||
|- server.js
|
||
```
|
||
|
||
访问插件管理器界面,查看刚添加的插件,默认地址为 http://localhost:13000/admin/pm/list/local/
|
||
|
||
<img src="https://nocobase.oss-cn-beijing.aliyuncs.com/b04d16851fc1bbc2796ecf8f9bc0c3f4.png" />
|
||
|
||
如果创建的插件未在插件管理器里显示,可以通过 `pm add` 命令手动添加
|
||
|
||
```bash
|
||
yarn pm add @my-project/plugin-hello
|
||
```
|
||
|
||
## 编写插件
|
||
|
||
查看 `packages/plugins/@my-project/plugin-hello/src/server/plugin.ts` 文件,并修改为:
|
||
|
||
```ts
|
||
import { InstallOptions, Plugin } from '@nocobase/server';
|
||
|
||
export class PluginHelloServer extends Plugin {
|
||
afterAdd() {}
|
||
|
||
beforeLoad() {}
|
||
|
||
async load() {
|
||
this.db.collection({
|
||
name: 'hello',
|
||
fields: [{ type: 'string', name: 'name' }],
|
||
});
|
||
this.app.acl.allow('hello', '*');
|
||
}
|
||
|
||
async install(options?: InstallOptions) {}
|
||
|
||
async afterEnable() {}
|
||
|
||
async afterDisable() {}
|
||
|
||
async remove() {}
|
||
}
|
||
|
||
export default PluginHelloServer;
|
||
```
|
||
|
||
## 激活插件
|
||
|
||
**通过命令操作**
|
||
|
||
```bash
|
||
yarn pm enable @my-project/plugin-hello
|
||
```
|
||
|
||
**通过界面操作**
|
||
|
||
访问插件管理器界面,查看刚添加的插件,点击激活。
|
||
插件管理器页面默认为 http://localhost:13000/admin/pm/list/local/
|
||
|
||
<img src="https://nocobase.oss-cn-beijing.aliyuncs.com/7b7df26a8ecc32bb1ebc3f99767ff9f9.png" />
|
||
|
||
备注:插件激活时,会自动创建刚才编辑插件配置的 hello 表。
|
||
|
||
## 调试插件
|
||
|
||
如果应用未启动,需要先启动应用
|
||
|
||
```bash
|
||
# for development
|
||
yarn dev
|
||
|
||
# for production
|
||
yarn build
|
||
yarn start
|
||
```
|
||
|
||
向插件的 hello 表里插入数据
|
||
|
||
```bash
|
||
curl --location --request POST 'http://localhost:13000/api/hello:create' \
|
||
--header 'Content-Type: application/json' \
|
||
--data-raw '{
|
||
"name": "Hello world"
|
||
}'
|
||
```
|
||
|
||
查看 hello 表数据
|
||
|
||
```bash
|
||
curl --location --request GET 'http://localhost:13000/api/hello:list'
|
||
```
|
||
|
||
## 构建并打包插件
|
||
|
||
```bash
|
||
yarn build plugins/@my-project/plugin-hello --tar
|
||
|
||
# 分步骤
|
||
yarn build plugins/@my-project/plugin-hello
|
||
yarn nocobase tar plugins/@my-project/plugin-hello
|
||
```
|
||
|
||
打包的插件默认保存路径为 `storage/tar/@my-project/plugin-hello.tar.gz`
|
||
|
||
## 上传至其他 NocoBase 应用
|
||
|
||
仅 v0.14 及以上版本支持
|
||
|
||
<img src="https://nocobase.oss-cn-beijing.aliyuncs.com/8aa8a511aa8c1e87a8f7ee82cf8a1359.gif" />
|