mirror of
https://github.com/nocobase/nocobase
synced 2024-11-15 23:26:38 +00:00
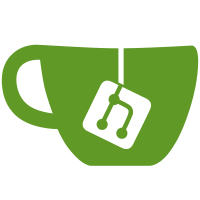
* refactor: change moment to dayjs * refactor: remove antd css * refactor: change @formily/antd to @formily/antd-v5 * chore: add dep * chore: upgrade babel/core and typescript * refactor: rename moment to dayjs * fix(dayjs): add plugins * refactor: fix type errors * refactor: change default export to named export * chore: upgrade ts-loader * refactor: rename moment to dayjs * refactor: fix type errors * chore: upgrade deps for build * fix: fix build errors * fix: add antd reset css * fix: fix build error * chore: add __builtins__ * chore: optimize genStyleHook * refactor(Calendar): less to css-in-js * refactor(acl): less to css-in-js * refactor(board): less to css-in-js * chore: add antd-style * refactor(acl): use antd-style * refactor(board): use antd-style * refactor: schema-initializer * refactor: refactor genStyleHook * refactor: kanban * refactor: filter * refactor: upload * refactor: markdown * refactor: rename className to componentCls * refactor: rich-text * style: fix style * fix: fix merge error * chore: update yarn.lock * chore: upgrade formily * style: fix pageHeader * style: fix add button style * style: fix header menu color * chore: update yarn.lock * chore: upgrade deps * test: fix tests * test: fix tests * fix: fix build error * fix: fix style of plugin doc * fix: fix tests * fix: fix drag bug * refactor: remove useless code * fix: fix Modal style (T-621) * fix: fix box-shadow of subMenu (T-622) * fix: fix style of linkage rules (T-623) * fix: fix style of DataTemplate * fix: fix style of variable (T-620) * chore: update yarn.lock * fix: avoid test failed * test: fix error * chore: update yarn.lock * test: fix error * test(dayjs): fix error * fix: should delay show menu to avoid the menu not hidden * test: skip failure test * fix(mouseEnterDelay): change default value from 100 to 150 * test: avoid failed * refactor: rename component names * chore: optimize types * chore: lock antd version * fix: fix build * fix: fix build * fix: layout bg color use variable * fix: fix style of buttons * feat: remove theme config * fix(calendar): fix style * fix(mobile-client): fix dialog style * fix: fix test * refactor: make code gooder * chore: change code * fix: fix T-847 * fix: fix T-845 * fix: display block * fix: danger button * refactor: make tester better * fix: change moment to dayjs * fix: build error * fix: import dayjs/plugin/isSameOrBefore * refactor: downgrade @testing-library/react to fix warning * fix: fix CI * fix: upgrade deps to fix build * fix: fix test * fix: skip some filed tests to avoid error * fix: fix build errors that maked by merge code * refactor: remove moment * fix: error * feat: update doc --------- Co-authored-by: chenos <chenlinxh@gmail.com>
3.1 KiB
3.1 KiB
Collection Manager
import React from 'react';
import { observer, ISchema, useForm } from '@formily/react';
import {
SchemaComponent,
SchemaComponentProvider,
Form,
Action,
CollectionProvider,
CollectionField,
} from '@nocobase/client';
import { FormItem, Input } from '@formily/antd-v5';
export default observer(() => {
const collection = {
name: 'tests',
fields: [
{
type: 'string',
name: 'title1',
interface: 'input',
uiSchema: {
title: 'Title1',
type: 'string',
'x-component': 'Input',
required: true,
description: 'description1',
} as ISchema,
},
{
type: 'string',
name: 'title2',
interface: 'input',
uiSchema: {
title: 'Title2',
type: 'string',
'x-component': 'Input',
description: 'description',
default: 'ttt',
},
},
{
type: 'string',
name: 'title3',
},
],
};
const schema: ISchema = {
type: 'object',
properties: {
form1: {
type: 'void',
'x-component': 'Form',
properties: {
// 字段 title1 直接使用全局提供的 uiSchema
title1: {
'x-component': 'CollectionField',
'x-decorator': 'FormItem',
default: '111',
},
// 等同于
// title1: {
// type: 'string',
// title: 'Title',
// required: true,
// 'x-component': 'Input',
// 'x-decorator': 'FormItem',
// },
title2: {
'x-component': 'CollectionField',
'x-decorator': 'FormItem',
title: 'Title4', // 覆盖全局已定义的 Title2
required: true, // 扩展的配置参数
description: 'description4',
},
// 等同于
// title2: {
// type: 'string',
// title: 'Title22',
// required: true,
// 'x-component': 'Input',
// 'x-decorator': 'FormItem',
// },
// 字段 title3 没有提供 uiSchema,自行处理
title3: {
'x-component': 'Input',
'x-decorator': 'FormItem',
title: 'Title3',
required: true,
},
action1: {
// type: 'void',
'x-component': 'Action',
title: 'Submit',
'x-component-props': {
type: 'primary',
useAction: '{{ useSubmit }}',
},
},
},
},
},
};
const useSubmit = () => {
const form = useForm();
return {
async run() {
form.submit(() => {
console.log(form.values);
});
},
};
};
return (
<SchemaComponentProvider scope={{ useSubmit }} components={{ Action, Form, CollectionField, Input, FormItem }}>
<CollectionProvider collection={collection}>
<SchemaComponent schema={schema} />
</CollectionProvider>
</SchemaComponentProvider>
);
});