mirror of
https://github.com/nocobase/nocobase
synced 2024-11-15 18:37:04 +00:00
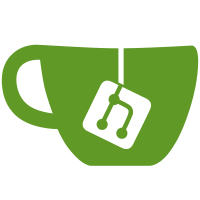
* Turkish language created for Docs. Belgeler için türkçe dil desteği oluşturuldu. * Turkish docs fix
1.7 KiB
1.7 KiB
UI Routing
NocoBase Client's Router is based on React Router and can be configured via <RouteSwitch routes={[]} />
to configure ui routes with the following example.
/**
* defaultShowCode: true
*/
import React from 'react';
import { Link, MemoryRouter as Router } from 'react-router-dom';
import { RouteRedirectProps, RouteSwitchProvider, RouteSwitch } from '@nocobase/client';
const Home = () => <h1>Home</h1>;
const About = () => <h1>About</h1>;
const routes: RouteRedirectProps[] = [
{
type: 'route',
path: '/',
exact: true,
component: 'Home',
},
{
type: 'route',
path: '/about',
component: 'About',
},
];
export default () => {
return (
<RouteSwitchProvider components={{ Home, About }}>
<Router initialEntries={['/']}>
<Link to={'/'}>Home</Link>, <Link to={'/about'}>About</Link>
<RouteSwitch routes={routes} />
</Router>
</RouteSwitchProvider>
);
};
In a full NocoBase application, the Route can be extended in a similar way as follows.
import { RouteSwitchContext } from '@nocobase/client';
import React, { useContext } from 'react';
const HelloWorld = () => {
return <div>Hello ui router</div>;
};
export default React.memo((props) => {
const ctx = useContext(RouteSwitchContext);
ctx.routes.push({
type: 'route',
path: '/hello-world',
component: HelloWorld,
});
return <RouteSwitchContext.Provider value={ctx}>{props.children}</RouteSwitchContext.Provider>;
});
See packages/samples/custom-page for the full example